前回までの記事
開発環境
環境 | バージョン |
---|---|
MacBook Air | Monterey 12.2.1 |
Docker | 20.10.7 |
PHP | 8.1 |
composer | 2.2.9 |
Laravel | 9.5.1 |
apache | 2.4 |
MySQL | 8.0 |
phpmyadmin | – |
npm | 8.5 |
node | 12.22.11 |
React | 17 |
TailwindCSS | 3 |
Laravel/breeze | ログイン認証機能で使用 ※Laravel8以降の場合に使用可。 Laravel6や7系はreact –authとか使います。 |
開発+α
◆dockerコンテナの情報を確認する
→docker ps
◆dockerのphpコンテナに入る
→docker exec -it コンテナIDまたはNAMES bash
◆var/www# はphpコンテナ内を表します。php artisanやnpm run devなどはphpコンテナ内で実行しましょう。
◆tailwindcssでスタイルを変更する前に
→npm run watchを実行していると開発が楽。
※ある程度スタイルが整ったら、npm run devでビルドしましょう。
すると、不要なcssを削除してくれるのでcssファイルが軽くなります。
◆スタイルが適用されないときは….
npm run devで一旦ビルドしてみるか、スーパーリロードでキャッシュの削除をしてみましょう。
- 記事をゴミ箱に移動(論理削除)
- 記事をゴミ箱から復元する
- 記事を完全に削除する(物理削除)
最終的なゴール
投稿リストからTrashをクリック

記事をゴミ箱に移動させ、投稿リストの画面上からは消える(論理削除なので、DB上からはデータ消えていない。delete_flgを0→1に更新するだけ。)

記事を復元するをクリック→記事を投稿リストに戻す(delete_flgを1→0に更新)、deleteをクリックすると記事を完全に削除する(物理削除)
また、記事をゴミ箱に移動、復元、削除するときは確認ダイアログを出す。
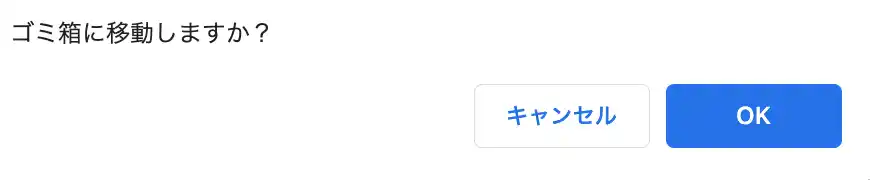

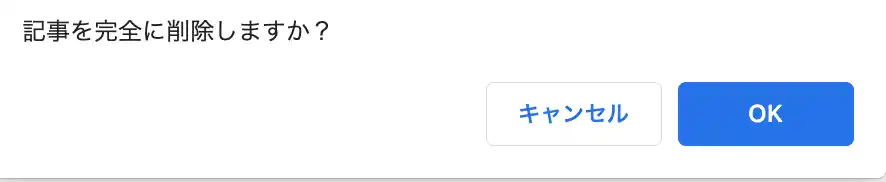
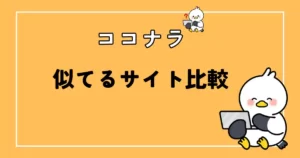
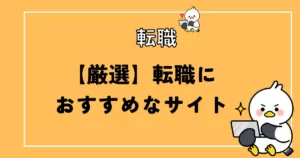
休日で空いた時間の暇つぶしを探せるアプリを公開しています。
ゴミ箱機能を実装する
ゴミ箱機能とはなんぞや?って話ですが、投稿記事一覧の画面上から記事をなくすけど、データとしては消さないようにするってことです。
ゴミ箱に移動した記事は、データとしては消していないので、記事を復元したりできます。
これは論理削除で実現できますが、postsテーブルの構造は以下のようになっているので、delete_flgが1ならば論理削除(つまり記事をゴミ箱にポイする)と判断します。
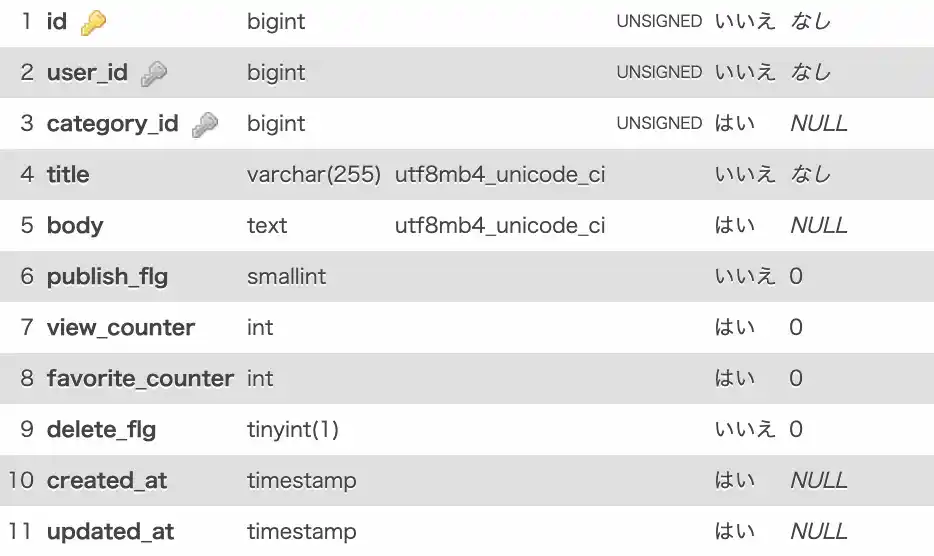
記事を完全に削除する場合(物理削除)は、postsテーブルにあるデータごと削除するので、画面上からもDB上からも削除するようにします。
ゴミ箱機能は、ざっと
- ゴミ箱にある記事一覧
- 投稿一覧からTrashをクリックした時にゴミ箱へ移動させる
- ゴミ箱から記事を復元する
- ゴミ箱にある記事を完全に削除する
といくつか機能があるので、新たにTrashコントローラーを作成し、そこにロジックを書いていきましょう。※Postコントロラーに書いてもいいけど、めっちゃ肥大化するためおすすめしない。
Trashコントローラーを作成する
ゴミ箱機能を処理するTrashコントローラーをartisanコマンドで作成します。
/var/www# php artisan make:controller User/TrashController
Controller created successfully.
src/app/Http/Controllers/User/TrashController.phpが作成されます。とりあえずPostコントローラーみたいに以下のようにモデルにアクセスできるようにはしておきます。
過去記事:モデルにDBロジックを書いてファットコントローラーを避ける
<?php
namespace App\Http\Controllers\User;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use App\Models\Post;
use App\Models\Category;
class TrashController extends Controller
{
private $post;
private $category;
public function __construct()
{
$this->post = new Post();
$this->category = new Category();
}
}
ゴミ箱一覧のルーティングパスを追加する
ルーティングを追加します。※冒頭のTrashControllerの読み込みを忘れずに!!
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TopController;
use App\Http\Controllers\User\PostController;
use App\Http\Controllers\User\TrashController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/dashboard', function () {
return view('dashboard');
})->middleware(['auth'])->name('dashboard');
require __DIR__.'/auth.php';
// 総合トップ
Route::get('/', [TopController::class, 'top'])
->name('top');
// 総合トップ記事詳細画面
Route::get('/article/{post_id}', [TopController::class, 'articleShow'])
->name('top.article.show');
// 総合トップカテゴリーごとの記事一覧
Route::get('/article/category/{category_id}', [TopController::class, 'articleCategory'])
->name('top.article.category');
// マイページトップ・投稿
Route::get('/user/{id}/index', [PostController::class, 'index'])
->name('user.index');
// 投稿登録画面
Route::get('/post/create', [PostController::class, 'create'])
->name('post.create');
// 投稿登録処理
Route::post('/post/store', [PostController::class, 'store'])
->name('post.store');
// 投稿詳細
Route::get('/post/show/{post_id}', [PostController::class, 'show'])
->name('post.show');
// 記事編集
Route::get('/post/edit/{post_id}', [PostController::class, 'edit'])
->name('post.edit');
// 記事更新
Route::post('/post/edit/{post_id}', [PostController::class, 'update'])
->name('post.update');
// 記事のゴミ箱
Route::get('/post/trash', [TrashController::class, 'trashList'])
->name('post.trash');
Trashコントローラーにゴミ箱一覧のアクションを追加する
TrashコントローラーにtrashListアクションを追加しましょう。
<?php
namespace App\Http\Controllers\User;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use App\Models\Post;
use App\Models\Category;
class TrashController extends Controller
{
private $post;
private $category;
public function __construct()
{
$this->post = new Post();
$this->category = new Category();
}
/**
* ゴミ箱一覧
*
* @return Response src/resources/views/user/list/trash.blade.phpを表示
*/
public function trashList()
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// ユーザーIDをもとに、論理削除されているdelete_flg=1のデータを取得
$trash_posts = $this->post->getTrashPostLists($user_id);
return view('user.list.trash', compact(
'user_id',
'trash_posts',
));
}
}
Postモデルにゴミ箱一覧を取得するロジックをかく
ゴミ箱にある投稿データを全て取得するgetTrashPostListsをPostモデルに追加します。
ポイントは、delete_flg=1のデータを取得することです。
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use App\Models\User;
use App\Models\Category;
use Illuminate\Support\Carbon;
/**
* 投稿モデル
*/
class Post extends Model
{
/**
* モデルに関連付けるテーブル
*
* @var string
*/
protected $table = 'posts';
/**
* 複数代入可能な属性
*
* @var array
*/
protected $fillable = [
'user_id',
'category_id',
'title',
'body',
'publish_flg',
'view_counter',
'favorite_counter',
'delete_flg',
'created_at',
'updated_at'
];
/**
* Userモデルとリレーション
*/
public function user()
{
return $this->belongsTo(User::class);
}
/**
* Categoryモデルとリレーション
*/
public function category()
{
return $this->belongsTo(Category::class);
}
/**
* 投稿データを全て取得し、最新更新日時順にソート
*/
public function getPostsSortByLatestUpdate()
{
$result = $this->where('publish_flg', 1)
->orderBy('updated_at', 'DESC')
->with('user')
->with('category')
->get();
return $result;
}
/**
* カテゴリーごとの記事を全て取得
*
* @param int $category_id カテゴリーID
*/
public function getPostByCategoryId($category_id)
{
$result = $this->where('category_id', $category_id)
->get();
return $result;
}
/**
* ユーザーIDに紐づいた投稿リストを全て取得する
*
* @param int $user_id ユーザーID
* @return Post
*/
public function getAllPostsByUserId($user_id)
{
$result = $this->where('user_id', $user_id)
->with('category')
->orderBy('updated_at', 'DESC')
->get();
return $result;
}
/**
* 下書き保存=>publish_flg=0
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToSaveDraft($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 公開=>publish_flg=1
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 予約公開=>publish_flg=2
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToReservationRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 投稿IDをもとにpostsテーブルから一意の投稿データを取得
*
* @param int $post_id 投稿ID
* @return object $result App\Models\Post
*/
public function feachPostDateByPostId($post_id)
{
$result = $this->find($post_id);
return $result;
}
/**
* 記事の更新処理
* 下書き保存=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToSaveDraft($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開=>publish_flg=1
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開予約=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToReservationRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
]);
$result->save();
return $result;
}
/**
* ゴミ箱一覧の記事を取得
*
* @param int $user_id ユーザーID
* @return object $result App\Models\Post
*/
public function getTrashPostLists($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 1],
])
->get();
return $result;
}
}
ゴミ箱一覧の画面を作成する
ゴミ箱一覧の画面を作成します。/resources/views/user/list/trash.blade.phpを新規で作成しましょう。
ちなみに、以下のソースで記事の復元やdeleteのformアクションのパスはこの後追加していくので今は空欄で大丈夫です。
{{-- src/resources/views/layouts/common.blade.php継承 --}}
@extends('layouts.common')
@include('user.parts.sidebar_user')
@section('content')
<div class="h-screen overflow-y-scroll">
<div class="px-4 sm:px-4">
<div class="flex justify-between">
<div class="text-2xl font-bold pt-7">ゴミ箱</div>
</div>
<div class="py-4">
<div class="overflow-x-auto">
<div class="inline-block min-w-full shadow rounded-lg overflow-hidden">
<table class="min-w-full leading-normal">
<thead>
<tr>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
タイトル
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
投稿ID
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
カテゴリー
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
ステータス
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
最終更新日時
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-left text-sm uppercase font-normal">
操作
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
PV数
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
お気に入り数
</th>
</tr>
</thead>
<tbody>
@foreach ($trash_posts as $post)
<tr>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm w-40">
<a href="{{ route('post.show', ['post_id' => $post->id]) }}" class="hover:underline">
<p class="text-left text-gray-900 whitespace-no-wrap">
{{ $post->title }}
</p>
</a>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->id }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
<span class="relative inline-block px-3 py-1 font-semibold text-white leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-green-500 rounded-full">
</span>
<span class="relative">
@if (isset($post->category_id))
{{ $post->category->category_name }}
@else
カテゴリーなし
@endif
</span>
</span>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
<form action="" method="POST">
@csrf
<button type="submit" class="mr-3 text-blue-700 whitespace-no-wrap underline">
投稿を復元する
</button>
</form>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->updated_at }}
</p>
</td>
<td class="px-5 py-5 mr-5 border-b border-gray-200 bg-white text-sm">
<div class="flex">
<form action="" method="POST">
@csrf
<button type="submit" class="underline text-red-700 whitespace-no-wrap">
delete
</button>
</form>
</div>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->view_counter }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->favorite_counter }}
</p>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
@endsection
マイページのサイドバー「ゴミ箱」をクリックしたら、/post/trashにアクセスして、ゴミ箱一覧画面が表示されるようにします。
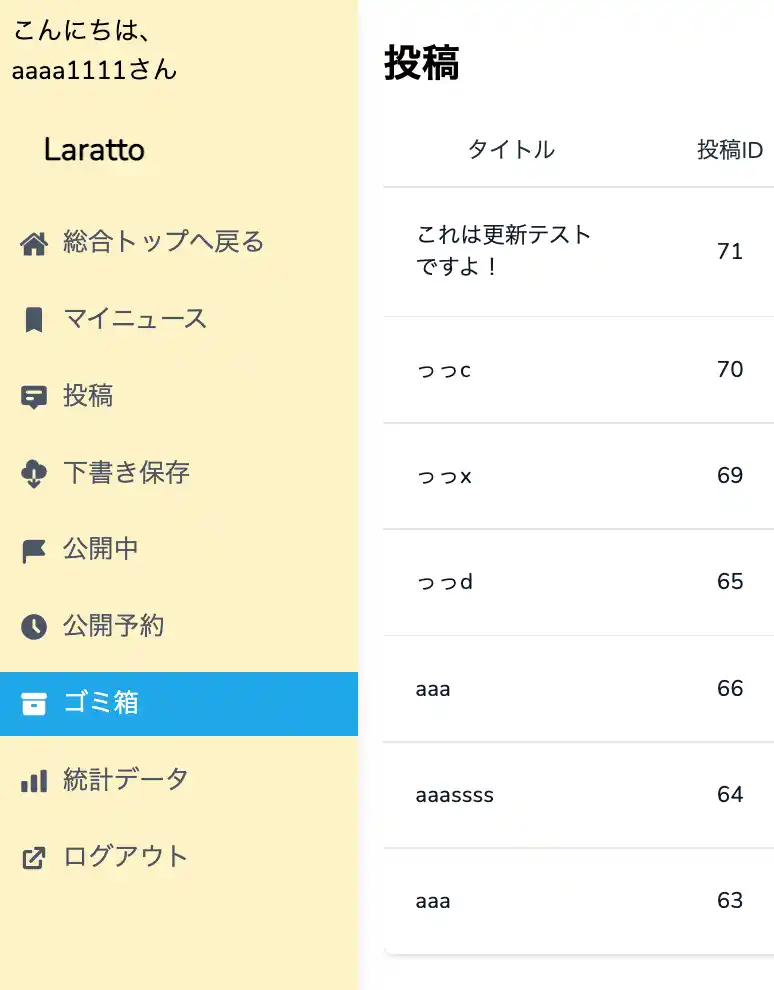
ゴミ箱一覧に遷移する流れです。
@section('sidebar')
<div class="h-screen hidden lg:block shadow-lg relative w-50">
<div class="bg-amber-100 h-full">
<div class="pt-3 pl-3">こんにちは、<br>{{ Auth::user()->name }}さん</div>
<div class="flex items-center justify-start pt-6 ml-8">
<p class="font-bold text-xl">
Laratto
</p>
</div>
<nav class="mt-6">
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="{{ route('top') }}">
<span class="text-left">
<svg width="20" height="20" fill="currentColor" viewBox="0 0 1792 1792" xmlns="http://www.w3.org/2000/svg">
<path d="M1472 992v480q0 26-19 45t-45 19h-384v-384h-256v384h-384q-26 0-45-19t-19-45v-480q0-1 .5-3t.5-3l575-474 575 474q1 2 1 6zm223-69l-62 74q-8 9-21 11h-3q-13 0-21-7l-692-577-692 577q-12 8-24 7-13-2-21-11l-62-74q-8-10-7-23.5t11-21.5l719-599q32-26 76-26t76 26l244 204v-195q0-14 9-23t23-9h192q14 0 23 9t9 23v408l219 182q10 8 11 21.5t-7 23.5z">
</path>
</svg>
</span>
<span class="mx-2 text-md font-normal">
総合トップへ戻る
</span>
</a>
</div>
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="#">
<span class="text-left">
<svg xmlns="http://www.w3.org/2000/svg" class="h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path d="M5 4a2 2 0 012-2h6a2 2 0 012 2v14l-5-2.5L5 18V4z" />
</svg>
</span>
<span class="mx-2 text-md font-normal">
マイニュース
</span>
</a>
</div>
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="{{ route('user.index', ['id' => Auth::user()->id]) }}">
<span class="text-left">
<svg xmlns="http://www.w3.org/2000/svg" class="h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path fill-rule="evenodd" d="M18 13V5a2 2 0 00-2-2H4a2 2 0 00-2 2v8a2 2 0 002 2h3l3 3 3-3h3a2 2 0 002-2zM5 7a1 1 0 011-1h8a1 1 0 110 2H6a1 1 0 01-1-1zm1 3a1 1 0 100 2h3a1 1 0 100-2H6z" clip-rule="evenodd" />
</svg>
</span>
<span class="mx-2 text-md font-normal">
投稿
</span>
</a>
</div>
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="#">
<span class="text-left">
<svg xmlns="http://www.w3.org/2000/svg" class="h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path fill-rule="evenodd" d="M2 9.5A3.5 3.5 0 005.5 13H9v2.586l-1.293-1.293a1 1 0 00-1.414 1.414l3 3a1 1 0 001.414 0l3-3a1 1 0 00-1.414-1.414L11 15.586V13h2.5a4.5 4.5 0 10-.616-8.958 4.002 4.002 0 10-7.753 1.977A3.5 3.5 0 002 9.5zm9 3.5H9V8a1 1 0 012 0v5z" clip-rule="evenodd" />
</svg>
</span>
<span class="mx-2 text-md font-normal">
下書き保存
</span>
</a>
</div>
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="#">
<span class="text-left">
<svg xmlns="http://www.w3.org/2000/svg" class="h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path fill-rule="evenodd" d="M10 18a8 8 0 100-16 8 8 0 000 16zm1-12a1 1 0 10-2 0v4a1 1 0 00.293.707l2.828 2.829a1 1 0 101.415-1.415L11 9.586V6z" clip-rule="evenodd" />
</svg>
</span>
<span class="mx-2 text-md font-normal">
公開予約
</span>
</a>
</div>
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="{{ route('post.trash') }}">
<span class="text-left">
<svg xmlns="http://www.w3.org/2000/svg" class="h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path d="M4 3a2 2 0 100 4h12a2 2 0 100-4H4z" />
<path fill-rule="evenodd" d="M3 8h14v7a2 2 0 01-2 2H5a2 2 0 01-2-2V8zm5 3a1 1 0 011-1h2a1 1 0 110 2H9a1 1 0 01-1-1z" clip-rule="evenodd" />
</svg>
</span>
<span class="mx-2 text-md font-normal">
ゴミ箱
</span>
</a>
</div>
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="#">
<span class="text-left">
<svg xmlns="http://www.w3.org/2000/svg" class="h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path d="M2 11a1 1 0 011-1h2a1 1 0 011 1v5a1 1 0 01-1 1H3a1 1 0 01-1-1v-5zM8 7a1 1 0 011-1h2a1 1 0 011 1v9a1 1 0 01-1 1H9a1 1 0 01-1-1V7zM14 4a1 1 0 011-1h2a1 1 0 011 1v12a1 1 0 01-1 1h-2a1 1 0 01-1-1V4z" />
</svg>
</span>
<span class="mx-2 text-md font-normal">
統計データ
</span>
</a>
</div>
<div>
<a class="flex items-center px-4 py-2 mt-2 text-gray-600 transition-colors duration-200 transform hover:bg-sky-500 hover:text-white"
href="#">
<span class="text-left">
<svg xmlns="http://www.w3.org/2000/svg" class="h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path d="M11 3a1 1 0 100 2h2.586l-6.293 6.293a1 1 0 101.414 1.414L15 6.414V9a1 1 0 102 0V4a1 1 0 00-1-1h-5z" />
<path d="M5 5a2 2 0 00-2 2v8a2 2 0 002 2h8a2 2 0 002-2v-3a1 1 0 10-2 0v3H5V7h3a1 1 0 000-2H5z" />
</svg>
</span>
<form action="{{ route('logout') }}" method="POST">
@csrf
<button>
<span class="mx-2 text-md font-normal">
ログアウト
</span>
</button>
</form>
</a>
</div>
</nav>
</div>
</div>
@endsection
投稿リストからゴミ箱に記事を移動する(論理削除)
ゴミ箱一覧のデータは取得し、画面の表示もできているかと思うので、ここからは投稿一覧からTrashをクリックしたら記事が論理削除されるようにします。
論理削除を実行する流れは、Trashをクリック→その記事のdelete_flgを1に更新するなので、メソッドはPOSTで送られます。
それでは、ルーティングを追加しましょう。
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TopController;
use App\Http\Controllers\User\PostController;
use App\Http\Controllers\User\TrashController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/dashboard', function () {
return view('dashboard');
})->middleware(['auth'])->name('dashboard');
require __DIR__.'/auth.php';
// 総合トップ
Route::get('/', [TopController::class, 'top'])
->name('top');
// 総合トップ記事詳細画面
Route::get('/article/{post_id}', [TopController::class, 'articleShow'])
->name('top.article.show');
// 総合トップカテゴリーごとの記事一覧
Route::get('/article/category/{category_id}', [TopController::class, 'articleCategory'])
->name('top.article.category');
// マイページトップ・投稿
Route::get('/user/{id}/index', [PostController::class, 'index'])
->name('user.index');
// 投稿登録画面
Route::get('/post/create', [PostController::class, 'create'])
->name('post.create');
// 投稿登録処理
Route::post('/post/store', [PostController::class, 'store'])
->name('post.store');
// 投稿詳細
Route::get('/post/show/{post_id}', [PostController::class, 'show'])
->name('post.show');
// 記事編集
Route::get('/post/edit/{post_id}', [PostController::class, 'edit'])
->name('post.edit');
// 記事更新
Route::post('/post/edit/{post_id}', [PostController::class, 'update'])
->name('post.update');
// 記事のゴミ箱
Route::get('/post/trash', [TrashController::class, 'trashList'])
->name('post.trash');
// 記事論理削除(ゴミ箱に移動)
Route::post('/post/trash/{post_id}', [TrashController::class, 'moveTrash'])
->name('post.move.trash');
投稿リストのdeleteは今となってはなんか記事を完全に削除するみたいなニュアンスがあって微妙なので、trashにします。このtrashを実行すると、論理削除されてゴミ箱に移動するようにしましょう。
before
<a class="ml-5 underline text-red-700 whitespace-no-wrap" href="#">
delete
</a>
after
{{-- src/resources/views/layouts/common.blade.php継承 --}}
@extends('layouts.common')
@include('user.parts.sidebar_user')
@section('content')
<div class="h-screen overflow-y-scroll">
<div class="px-4 sm:px-4">
<div class="flex justify-between">
<div class="text-2xl font-bold pt-7">投稿</div>
<div class="pt-4">
<a href="{{ route('post.create') }}">
<button class="bg-blue-500 text-white px-4 py-2 rounded-md text-1xl font-medium hover:bg-blue-700 transition duration-300">
新規追加
</button>
</a>
</div>
</div>
<div class="py-4">
<div class="overflow-x-auto">
<div class="inline-block min-w-full shadow rounded-lg overflow-hidden">
<table class="min-w-full leading-normal">
<thead>
<tr>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
タイトル
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
投稿ID
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
カテゴリー
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
ステータス
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
最終更新日時
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-left text-sm uppercase font-normal">
操作
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
PV数
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
お気に入り数
</th>
</tr>
</thead>
<tbody>
@foreach ($posts as $post)
<tr>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm w-40">
<a href="{{ route('post.show', ['post_id' => $post->id]) }}" class="hover:underline">
<p class="text-left text-gray-900 whitespace-no-wrap">
{{ $post->title }}
</p>
</a>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->id }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
<span class="relative inline-block px-3 py-1 font-semibold text-white leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-green-500 rounded-full">
</span>
<span class="relative">
@if (isset($post->category_id))
{{ $post->category->category_name }}
@else
カテゴリーなし
@endif
</span>
</span>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
@if ($post->publish_flg === 0)
<span class="relative inline-block px-3 py-1 font-semibold text-blue-900 leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-blue-200 opacity-50 rounded-full">
</span>
<span class="relative">
下書き保存
</span>
</span>
@elseif ($post->publish_flg === 1)
<span class="relative inline-block px-3 py-1 font-semibold text-green-900 leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-green-200 opacity-50 rounded-full">
</span>
<span class="relative">公開済み</span>
</span>
@elseif ($post->publish_flg === 2)
<span class="relative inline-block px-3 py-1 font-semibold text-amber-900 leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-amber-200 opacity-50 rounded-full">
</span>
<span class="relative">予約公開</span>
</span>
@else
<span class="relative inline-block px-3 py-1 font-semibold text-green-900 leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-green-200 opacity-50 rounded-full">
</span>
<span class="relative">下書き保存</span>
</span>
@endif
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->updated_at }}
</p>
</td>
<td class="px-5 py-5 mr-5 border-b border-gray-200 bg-white text-sm">
<div class="flex">
<a class="mr-3 text-blue-700 whitespace-no-wrap underline" href="{{ route('post.edit', ['post_id' => $post->id]) }}">
Edit
</a>
<form action="{{ route('post.move.trash', ['post_id' => $post->id]) }}" method="POST" onSubmit="return is_move_trash()">
@csrf
<button type="submit" class="underline text-red-700 whitespace-no-wrap">
Trash
</button>
</form>
</div>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->view_counter }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->favorite_counter }}
</p>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
<script>
function is_move_trash() {
const moveTarashMessage = 'ゴミ箱に移動しますか?';
const cancelMessage = 'キャンセルされました';
// trashをクリックした時に確認ダイアログを表示。OKで実行、キャンセルで実行しない。
if(window.confirm(moveTarashMessage)){
return true;
} else {
window.alert(cancelMessage);
return false;
}
}
</script>
@endsection
キャンセル押すと、イベントが実行されないので記事はゴミ箱に移動せず、OKを押すとイベントが実行されて記事がゴミ箱に移動するようになる予定(これからゴミ箱に移動させるロジックを作るので今はエラー出ると思います)
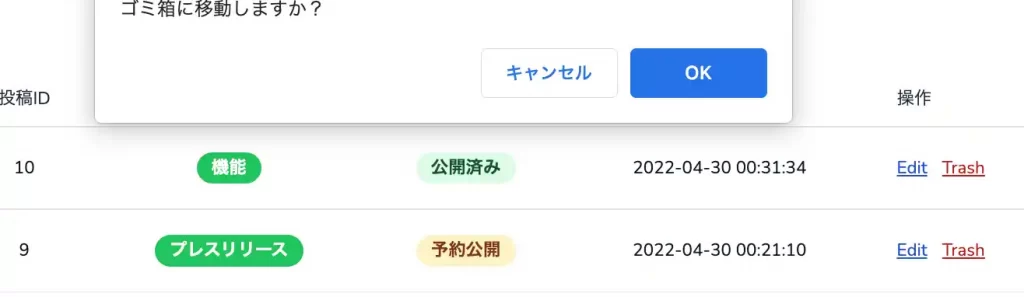
Trashコントローラーに記事をゴミ箱に移動する処理を書く
Trashを押下時に記事をゴミ箱に移動させるmoveTrashアクションをTrashコントローラーに追加します。
<?php
namespace App\Http\Controllers\User;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use App\Models\Post;
use App\Models\Category;
class TrashController extends Controller
{
private $post;
private $category;
public function __construct()
{
$this->post = new Post();
$this->category = new Category();
}
/**
* ゴミ箱一覧
*
* @return Response src/resources/views/user/list/trash.blade.phpを表示
*/
public function trashList()
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// ユーザーIDをもとに、論理削除されているdelete_flg=1のデータを取得
$trash_posts = $this->post->getTrashPostLists($user_id);
return view('user.list.trash', compact(
'user_id',
'trash_posts',
));
}
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param int $post_id 投稿ID
*/
public function moveTrash($post_id)
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// 投稿IDをもとに特定の投稿データを取得
$post = $this->post->feachPostDateByPostId($post_id);
// 記事を論理削除(ゴミ箱に移動)
$trashPost = $this->post->moveTrashPostData($post);
// マイページ投稿リストにリダイレクト
return to_route('user.index', ['id' => $user_id]);
}
}
Postモデルにゴミ箱に記事を移動させる(論理削除)ロジックをかく
記事を論理削除させて、ゴミ箱に移動させるロジック、moveTrashPostDataをPostモデルに追加します。
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use App\Models\User;
use App\Models\Category;
use Illuminate\Support\Carbon;
/**
* 投稿モデル
*/
class Post extends Model
{
/**
* モデルに関連付けるテーブル
*
* @var string
*/
protected $table = 'posts';
/**
* 複数代入可能な属性
*
* @var array
*/
protected $fillable = [
'user_id',
'category_id',
'title',
'body',
'publish_flg',
'view_counter',
'favorite_counter',
'delete_flg',
'created_at',
'updated_at'
];
/**
* Userモデルとリレーション
*/
public function user()
{
return $this->belongsTo(User::class);
}
/**
* Categoryモデルとリレーション
*/
public function category()
{
return $this->belongsTo(Category::class);
}
/**
* 投稿データを全て取得し、最新更新日時順にソート
*/
public function getPostsSortByLatestUpdate()
{
$result = $this->where('publish_flg', 1)
->orderBy('updated_at', 'DESC')
->with('user')
->with('category')
->get();
return $result;
}
/**
* カテゴリーごとの記事を全て取得
*
* @param int $category_id カテゴリーID
*/
public function getPostByCategoryId($category_id)
{
$result = $this->where('category_id', $category_id)
->get();
return $result;
}
/**
* ユーザーIDに紐づいた投稿リストを全て取得する
*
* @param int $user_id ユーザーID
* @return Post
*/
public function getAllPostsByUserId($user_id)
{
$result = $this->where('user_id', $user_id)
->with('category')
->orderBy('updated_at', 'DESC')
->get();
return $result;
}
/**
* 下書き保存=>publish_flg=0
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToSaveDraft($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 公開=>publish_flg=1
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 予約公開=>publish_flg=2
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToReservationRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 投稿IDをもとにpostsテーブルから一意の投稿データを取得
*
* @param int $post_id 投稿ID
* @return object $result App\Models\Post
*/
public function feachPostDateByPostId($post_id)
{
$result = $this->find($post_id);
return $result;
}
/**
* 記事の更新処理
* 下書き保存=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToSaveDraft($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開=>publish_flg=1
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開予約=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToReservationRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
]);
$result->save();
return $result;
}
/**
* ゴミ箱一覧の記事を取得
*
* @param int $user_id ユーザーID
* @return object $result App\Models\Post
*/
public function getTrashPostLists($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 1],
])
->get();
return $result;
}
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function moveTrashPostData($post)
{
$result = $post->fill([
'delete_flg' => 1
]);
$result->save();
return $result;
}
}
これで投稿リストの記事のTrashをクリックして確認ダイアログのOKをクリックすると、その記事のdelete_flgが1に更新されて論理削除されたことになります。
流れとしては、記事一覧でTrashをクリック
↓
その記事のdelete_flgを1に更新する※できた
↓
記事一覧や総合トップの記事一覧取得は、delete_flg=0のデータを取得するようにしているので、どちらの画面からも記事は非表示になる※まだ
↓
記事がゴミ箱一覧画面に追加される※できた
↓
ゴミ箱に移動した記事は、記事のステータスは下書き保存にする
↓
ゴミ箱一覧の記事は、delete_flg=1のデータのみ取得して表示
データベースを確認してみると、delete_flgが1になっていることが確認できますよ〜。
ただ、今のままだと投稿リストに記事が残ったままです。
この原因は、記事の一覧を取得する際に、delete_flgが1のものは表示しないように制御していないからです。なので、whereを使って、delete_flgが0のデータだけ取得するように修正します。
マイページの投稿リストだけでなく、総合トップの記事一覧取得の際にもdelete_flgが1のデータは表示したくないのでそれも加えておきましょう。
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use App\Models\User;
use App\Models\Category;
use Illuminate\Support\Carbon;
/**
* 投稿モデル
*/
class Post extends Model
{
/**
* モデルに関連付けるテーブル
*
* @var string
*/
protected $table = 'posts';
/**
* 複数代入可能な属性
*
* @var array
*/
protected $fillable = [
'user_id',
'category_id',
'title',
'body',
'publish_flg',
'view_counter',
'favorite_counter',
'delete_flg',
'created_at',
'updated_at'
];
/**
* Userモデルとリレーション
*/
public function user()
{
return $this->belongsTo(User::class);
}
/**
* Categoryモデルとリレーション
*/
public function category()
{
return $this->belongsTo(Category::class);
}
/**
* 投稿データを全て取得し、最新更新日時順にソート
*/
public function getPostsSortByLatestUpdate()
{
$result = $this->where([
['publish_flg', 1],
['delete_flg', 0],
])
->orderBy('updated_at', 'DESC')
->with('user')
->with('category')
->get();
return $result;
}
/**
* カテゴリーごとの記事を全て取得
*
* @param int $category_id カテゴリーID
*/
public function getPostByCategoryId($category_id)
{
$result = $this->where([
['category_id', $category_id],
['delete_flg', 0],
])
->get();
return $result;
}
/**
* ユーザーIDに紐づいた投稿リストを全て取得する
*
* @param int $user_id ユーザーID
* @return Post
*/
public function getAllPostsByUserId($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 0],
])
->with('category')
->orderBy('updated_at', 'DESC')
->get();
return $result;
}
/**
* 下書き保存=>publish_flg=0
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToSaveDraft($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 公開=>publish_flg=1
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 予約公開=>publish_flg=2
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToReservationRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 投稿IDをもとにpostsテーブルから一意の投稿データを取得
*
* @param int $post_id 投稿ID
* @return object $result App\Models\Post
*/
public function feachPostDateByPostId($post_id)
{
$result = $this->find($post_id);
return $result;
}
/**
* 記事の更新処理
* 下書き保存=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToSaveDraft($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開=>publish_flg=1
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開予約=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToReservationRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
]);
$result->save();
return $result;
}
/**
* ゴミ箱一覧の記事を取得
*
* @param int $user_id ユーザーID
* @return object $result App\Models\Post
*/
public function getTrashPostLists($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 1],
])
->get();
return $result;
}
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function moveTrashPostData($post)
{
$result = $post->fill([
'delete_flg' => 1
]);
$result->save();
return $result;
}
}
ゴミ箱で記事の復元をする
ゴミ箱に記事を移動させる論理削除はできたと思うので、今度は記事を復元させましょう。
流れとしては、こんな感じです。うん、楽勝。
ゴミ箱一覧の該当記事の記事を復元するをクリック
↓
delete_flgを1→0に更新
記事を復元するためのルーティングを追加する
記事を復元する処理は、delete_flgを1→0に更新するなので、メソッドはPOSTになります。
ルーティングを追加します。
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TopController;
use App\Http\Controllers\User\PostController;
use App\Http\Controllers\User\TrashController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/dashboard', function () {
return view('dashboard');
})->middleware(['auth'])->name('dashboard');
require __DIR__.'/auth.php';
// 総合トップ
Route::get('/', [TopController::class, 'top'])
->name('top');
// 総合トップ記事詳細画面
Route::get('/article/{post_id}', [TopController::class, 'articleShow'])
->name('top.article.show');
// 総合トップカテゴリーごとの記事一覧
Route::get('/article/category/{category_id}', [TopController::class, 'articleCategory'])
->name('top.article.category');
// マイページトップ・投稿
Route::get('/user/{id}/index', [PostController::class, 'index'])
->name('user.index');
// 投稿登録画面
Route::get('/post/create', [PostController::class, 'create'])
->name('post.create');
// 投稿登録処理
Route::post('/post/store', [PostController::class, 'store'])
->name('post.store');
// 投稿詳細
Route::get('/post/show/{post_id}', [PostController::class, 'show'])
->name('post.show');
// 記事編集
Route::get('/post/edit/{post_id}', [PostController::class, 'edit'])
->name('post.edit');
// 記事更新
Route::post('/post/edit/{post_id}', [PostController::class, 'update'])
->name('post.update');
// 記事のゴミ箱
Route::get('/post/trash', [TrashController::class, 'trashList'])
->name('post.trash');
// 記事論理削除(ゴミ箱に移動)
Route::post('/post/trash/{post_id}', [TrashController::class, 'moveTrash'])
->name('post.move.trash');
// 記事の復元(ゴミ箱から投稿リストに戻す)
Route::post('/post/restore/{post_id}', [TrashController::class, 'restore'])
->name('post.restore');
ゴミ箱で記事を復元するをクリックした時に、確認ダイアログを表示させる+空欄だったformアクションにrestoreを追加していきます。
{{-- src/resources/views/layouts/common.blade.php継承 --}}
@extends('layouts.common')
@include('user.parts.sidebar_user')
@section('content')
<div class="h-screen overflow-y-scroll">
<div class="px-4 sm:px-4">
<div class="flex justify-between">
<div class="text-2xl font-bold pt-7">ゴミ箱</div>
</div>
<div class="py-4">
<div class="overflow-x-auto">
<div class="inline-block min-w-full shadow rounded-lg overflow-hidden">
<table class="min-w-full leading-normal">
<thead>
<tr>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
タイトル
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
投稿ID
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
カテゴリー
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
ステータス
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
最終更新日時
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-left text-sm uppercase font-normal">
操作
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
PV数
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
お気に入り数
</th>
</tr>
</thead>
<tbody>
@foreach ($trash_posts as $post)
<tr>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm w-40">
<a href="{{ route('post.show', ['post_id' => $post->id]) }}" class="hover:underline">
<p class="text-left text-gray-900 whitespace-no-wrap">
{{ $post->title }}
</p>
</a>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->id }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
<span class="relative inline-block px-3 py-1 font-semibold text-white leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-green-500 rounded-full">
</span>
<span class="relative">
@if (isset($post->category_id))
{{ $post->category->category_name }}
@else
カテゴリーなし
@endif
</span>
</span>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
<form action="{{ route('post.restore', ['post_id' => $post->id]) }}" method="POST" onSubmit="return is_restore_check()">
@csrf
<button type="submit" class="mr-3 text-blue-700 whitespace-no-wrap underline">
投稿を復元する
</button>
</form>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->updated_at }}
</p>
</td>
<td class="px-5 py-5 mr-5 border-b border-gray-200 bg-white text-sm">
<div class="flex">
<form action="" method="POST">
@csrf
<button type="submit" class="underline text-red-700 whitespace-no-wrap">
delete
</button>
</form>
</div>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->view_counter }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->favorite_counter }}
</p>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
<script>
function is_restore_check() {
const restore = '記事を復元しますか?';
const cancel = 'キャンセルされました';
// 記事を復元するをクリックした時に確認ダイアログを表示。OKで実行、キャンセルで実行しない。
if(window.confirm(restore)){
return true;
} else {
window.alert(cancel);
return false;
}
}
</script>
@endsection
これでひとまず、
- 記事を復元するをクリックした時に「記事を復元する?」の確認ダイアログが表示
- キャンセルを押すとイベントが実行されない
- OKを押すとイベントが実行される
- まだ、Trashコントローラーにrestoreアクションを追加していないので、エラーは出る
- なので、この後、restoreアクションを追加する
こんな感じになります。

Trashコントローラーに記事の復元アクションを追加する
Trashコントローラーに記事を復元するrestoreアクションを追加します。
<?php
namespace App\Http\Controllers\User;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use App\Models\Post;
use App\Models\Category;
class TrashController extends Controller
{
private $post;
private $category;
public function __construct()
{
$this->post = new Post();
$this->category = new Category();
}
/**
* ゴミ箱一覧
*
* @return Response src/resources/views/user/list/trash.blade.phpを表示
*/
public function trashList()
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// ユーザーIDをもとに、論理削除されているdelete_flg=1のデータを取得
$trash_posts = $this->post->getTrashPostLists($user_id);
return view('user.list.trash', compact(
'user_id',
'trash_posts',
));
}
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param int $post_id 投稿ID
*/
public function moveTrash($post_id)
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// 投稿IDをもとに特定の投稿データを取得
$post = $this->post->feachPostDateByPostId($post_id);
// 記事を論理削除(ゴミ箱に移動)
$trashPost = $this->post->moveTrashPostData($post);
// マイページ投稿リストにリダイレクト
return to_route('user.index', ['id' => $user_id]);
}
/**
* 記事の復元
*
* @param int $post_id 投稿ID
*/
public function restore($post_id)
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// ユーザーIDをもとに、論理削除されているdelete_flg=1のデータを取得
$trash_posts = $this->post->getTrashPostLists($user_id);
// 投稿IDをもとに特定の投稿データを取得
$post = $this->post->feachPostDateByPostId($post_id);
// 記事の復元
$restorePost = $this->post->restorePostData($post);
// ゴミ箱にリダイレクト
return to_route('post.trash', compact(
'user_id',
'trash_posts',
));
}
}
まだ記事の復元を押しても、restorePostDataのロジックを作っていないので、以下のようにエラーが出ます。
Call to undefined method App\Models\Post::restorePostData()
PostモデルにrestorePostDataを作っていきましょう。
記事の復元をするためには、delete_flgを1→0に戻すだけでよさそうです。
delete_flgが0なら論理削除していない、1なら論理削除している(=ゴミ箱に記事を移動)かつ投稿リストや総合トップには記事を表示しない役割があります。
Postモデルに記事を復元するロジックをかく
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use App\Models\User;
use App\Models\Category;
use Illuminate\Support\Carbon;
/**
* 投稿モデル
*/
class Post extends Model
{
/**
* モデルに関連付けるテーブル
*
* @var string
*/
protected $table = 'posts';
/**
* 複数代入可能な属性
*
* @var array
*/
protected $fillable = [
'user_id',
'category_id',
'title',
'body',
'publish_flg',
'view_counter',
'favorite_counter',
'delete_flg',
'created_at',
'updated_at'
];
/**
* Userモデルとリレーション
*/
public function user()
{
return $this->belongsTo(User::class);
}
/**
* Categoryモデルとリレーション
*/
public function category()
{
return $this->belongsTo(Category::class);
}
/**
* 投稿データを全て取得し、最新更新日時順にソート
*/
public function getPostsSortByLatestUpdate()
{
$result = $this->where([
['publish_flg', 1],
['delete_flg', 0],
])
->orderBy('updated_at', 'DESC')
->with('user')
->with('category')
->get();
return $result;
}
/**
* カテゴリーごとの記事を全て取得
*
* @param int $category_id カテゴリーID
*/
public function getPostByCategoryId($category_id)
{
$result = $this->where([
['category_id', $category_id],
['delete_flg', 0],
])
->get();
return $result;
}
/**
* ユーザーIDに紐づいた投稿リストを全て取得する
*
* @param int $user_id ユーザーID
* @return Post
*/
public function getAllPostsByUserId($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 0],
])
->with('category')
->orderBy('updated_at', 'DESC')
->get();
return $result;
}
/**
* 下書き保存=>publish_flg=0
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToSaveDraft($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 公開=>publish_flg=1
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 予約公開=>publish_flg=2
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToReservationRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 投稿IDをもとにpostsテーブルから一意の投稿データを取得
*
* @param int $post_id 投稿ID
* @return object $result App\Models\Post
*/
public function feachPostDateByPostId($post_id)
{
$result = $this->find($post_id);
return $result;
}
/**
* 記事の更新処理
* 下書き保存=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToSaveDraft($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開=>publish_flg=1
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開予約=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToReservationRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
]);
$result->save();
return $result;
}
/**
* ゴミ箱一覧の記事を取得
*
* @param int $user_id ユーザーID
* @return object $result App\Models\Post
*/
public function getTrashPostLists($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 1],
])
->get();
return $result;
}
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function moveTrashPostData($post)
{
$result = $post->fill([
'delete_flg' => 1
]);
$result->save();
return $result;
}
/**
* 記事の復元
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function restorePostData($post)
{
$result = $post->fill([
'delete_flg' => 0
]);
$result->save();
return $result;
}
}
見ての通り、delete_flgを更新するぐらいなので、簡単っすね!
記事を完全に削除する(物理削除)
これまで、記事を論理削除してゴミ箱に移動させたり、復元させたりするために、delete_flgを更新する作業をしてきました。
これから行うのは、完全に記事を削除することで、論理削除ではなく物理削除です。
論理削除ではdelete_flgを1にして画面上は記事が削除されたことになっていますが、DBにはデータがありました。ただ、物理削除の場合は、DB上からも削除するという違いがあります。
ルーティングを追加します。
データを削除するので、メソッドはPOSTになります。
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TopController;
use App\Http\Controllers\User\PostController;
use App\Http\Controllers\User\TrashController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/dashboard', function () {
return view('dashboard');
})->middleware(['auth'])->name('dashboard');
require __DIR__.'/auth.php';
// 総合トップ
Route::get('/', [TopController::class, 'top'])
->name('top');
// 総合トップ記事詳細画面
Route::get('/article/{post_id}', [TopController::class, 'articleShow'])
->name('top.article.show');
// 総合トップカテゴリーごとの記事一覧
Route::get('/article/category/{category_id}', [TopController::class, 'articleCategory'])
->name('top.article.category');
// マイページトップ・投稿
Route::get('/user/{id}/index', [PostController::class, 'index'])
->name('user.index');
// 投稿登録画面
Route::get('/post/create', [PostController::class, 'create'])
->name('post.create');
// 投稿登録処理
Route::post('/post/store', [PostController::class, 'store'])
->name('post.store');
// 投稿詳細
Route::get('/post/show/{post_id}', [PostController::class, 'show'])
->name('post.show');
// 記事編集
Route::get('/post/edit/{post_id}', [PostController::class, 'edit'])
->name('post.edit');
// 記事更新
Route::post('/post/edit/{post_id}', [PostController::class, 'update'])
->name('post.update');
// 記事のゴミ箱
Route::get('/post/trash', [TrashController::class, 'trashList'])
->name('post.trash');
// 記事論理削除(ゴミ箱に移動)
Route::post('/post/trash/{post_id}', [TrashController::class, 'moveTrash'])
->name('post.move.trash');
// 記事の復元(ゴミ箱から投稿リストに戻す)
Route::post('/post/restore/{post_id}', [TrashController::class, 'restore'])
->name('post.restore');
// 記事を完全に削除
Route::post('/post/delete/{post_id}', [TrashController::class, 'delete'])
->name('post.delete');
ゴミ箱のdeleteをクリックした時に「記事を完全に削除するか?」の確認ダイアログを表示させ、空欄になっているformアクションにdeleteを追加します。
{{-- src/resources/views/layouts/common.blade.php継承 --}}
@extends('layouts.common')
@include('user.parts.sidebar_user')
@section('content')
<div class="h-screen overflow-y-scroll">
<div class="px-4 sm:px-4">
<div class="flex justify-between">
<div class="text-2xl font-bold pt-7">ゴミ箱</div>
</div>
<div class="py-4">
<div class="overflow-x-auto">
<div class="inline-block min-w-full shadow rounded-lg overflow-hidden">
<table class="min-w-full leading-normal">
<thead>
<tr>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
タイトル
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
投稿ID
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
カテゴリー
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
ステータス
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
最終更新日時
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-left text-sm uppercase font-normal">
操作
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
PV数
</th>
<th scope="col" class="px-5 py-3 bg-white border-b border-gray-200 text-gray-800 text-center text-sm uppercase font-normal">
お気に入り数
</th>
</tr>
</thead>
<tbody>
@foreach ($trash_posts as $post)
<tr>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm w-40">
<a href="{{ route('post.show', ['post_id' => $post->id]) }}" class="hover:underline">
<p class="text-left text-gray-900 whitespace-no-wrap">
{{ $post->title }}
</p>
</a>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->id }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
<span class="relative inline-block px-3 py-1 font-semibold text-white leading-tight">
<span aria-hidden="true" class="absolute inset-0 bg-green-500 rounded-full">
</span>
<span class="relative">
@if (isset($post->category_id))
{{ $post->category->category_name }}
@else
カテゴリーなし
@endif
</span>
</span>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm text-center">
<form action="{{ route('post.restore', ['post_id' => $post->id]) }}" method="POST" onSubmit="return is_restore_check()">
@csrf
<button type="submit" class="mr-3 text-blue-700 whitespace-no-wrap underline">
投稿を復元する
</button>
</form>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->updated_at }}
</p>
</td>
<td class="px-5 py-5 mr-5 border-b border-gray-200 bg-white text-sm">
<div class="flex">
<form action="{{ route('post.delete', ['post_id' => $post->id]) }}" method="POST" onSubmit="return is_delete_check()">
@csrf
<button type="submit" class="underline text-red-700 whitespace-no-wrap">
delete
</button>
</form>
</div>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->view_counter }}
</p>
</td>
<td class="px-5 py-5 border-b border-gray-200 bg-white text-sm">
<p class="text-center text-gray-900 whitespace-no-wrap">
{{ $post->favorite_counter }}
</p>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
<script>
function is_restore_check() {
const restore = '記事を復元しますか?';
const cancel = 'キャンセルされました';
// 記事を復元するをクリックした時に確認ダイアログを表示。OKで実行、キャンセルで実行しない。
if(window.confirm(restore)){
return true;
} else {
window.alert(cancel);
return false;
}
}
function is_delete_check() {
const deleteMessage = '記事を完全に削除しますか?';
const cancel = 'キャンセルされました';
// deleteをクリックした時に確認ダイアログを表示。OKでdelete実行、キャンセルでdelete実行しない。
if(window.confirm(deleteMessage)){
return true;
} else {
window.alert(cancel);
return false;
}
}
</script>
@endsection
キャンセルを押すとイベントは実行されず、OKを押すとイベントが実行されてTrashコントローラーのdeleteアクションが呼ばれます。
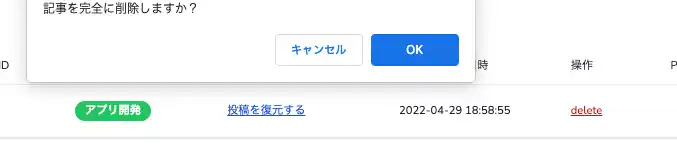
現段階では、まだdeleteアクションを作っていないので、エラー出ると思います。なので、deleteアクションをTrashコントローラーに追加しましょう。
Trashコントローラーに記事を削除するアクションを追加する
<?php
namespace App\Http\Controllers\User;
use App\Http\Controllers\Controller;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Auth;
use App\Models\Post;
use App\Models\Category;
class TrashController extends Controller
{
private $post;
private $category;
public function __construct()
{
$this->post = new Post();
$this->category = new Category();
}
/**
* ゴミ箱一覧
*
* @return Response src/resources/views/user/list/trash.blade.phpを表示
*/
public function trashList()
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// ユーザーIDをもとに、論理削除されているdelete_flg=1のデータを取得
$trash_posts = $this->post->getTrashPostLists($user_id);
return view('user.list.trash', compact(
'user_id',
'trash_posts',
));
}
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param int $post_id 投稿ID
*/
public function moveTrash($post_id)
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// 投稿IDをもとに特定の投稿データを取得
$post = $this->post->feachPostDateByPostId($post_id);
// 記事を論理削除(ゴミ箱に移動)
$trashPost = $this->post->moveTrashPostData($post);
// マイページ投稿リストにリダイレクト
return to_route('user.index', ['id' => $user_id]);
}
/**
* 記事の復元
*
* @param int $post_id 投稿ID
*/
public function restore($post_id)
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// ユーザーIDをもとに、論理削除されているdelete_flg=1のデータを取得
$trash_posts = $this->post->getTrashPostLists($user_id);
// 投稿IDをもとに特定の投稿データを取得
$post = $this->post->feachPostDateByPostId($post_id);
// 記事の復元
$restorePost = $this->post->restorePostData($post);
// ゴミ箱にリダイレクト
return to_route('post.trash', compact(
'user_id',
'trash_posts',
));
}
/**
* 記事をゴミ箱から削除(物理削除なので、完全にデータを削除する)
*
* @param int $post_id 投稿ID
*/
public function delete($post_id)
{
// ログインしているユーザー情報を取得
$user = Auth::user();
// ログインユーザー情報からユーザーIDを取得
$user_id = $user->id;
// ユーザーIDをもとに、論理削除されているdelete_flg=1のデータを取得
$trash_posts = $this->post->getTrashPostLists($user_id);
// 投稿IDをもとに特定の投稿データを取得
$post = $this->post->feachPostDateByPostId($post_id);
// 記事を物理削除(ゴミ箱からも削除)
$deletePost = $this->post->deletePostData($post);
// ゴミ箱にリダイレクト
return to_route('post.trash', compact(
'user_id',
'trash_posts',
));
}
}
Postモデルに記事を削除するロジックをかく
PostモデルにdeletePostDataのロジックを追加します。
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
use App\Models\User;
use App\Models\Category;
use Illuminate\Support\Carbon;
/**
* 投稿モデル
*/
class Post extends Model
{
/**
* モデルに関連付けるテーブル
*
* @var string
*/
protected $table = 'posts';
/**
* 複数代入可能な属性
*
* @var array
*/
protected $fillable = [
'user_id',
'category_id',
'title',
'body',
'publish_flg',
'view_counter',
'favorite_counter',
'delete_flg',
'created_at',
'updated_at'
];
/**
* Userモデルとリレーション
*/
public function user()
{
return $this->belongsTo(User::class);
}
/**
* Categoryモデルとリレーション
*/
public function category()
{
return $this->belongsTo(Category::class);
}
/**
* 投稿データを全て取得し、最新更新日時順にソート
*/
public function getPostsSortByLatestUpdate()
{
$result = $this->where([
['publish_flg', 1],
['delete_flg', 0],
])
->orderBy('updated_at', 'DESC')
->with('user')
->with('category')
->get();
return $result;
}
/**
* カテゴリーごとの記事を全て取得
*
* @param int $category_id カテゴリーID
*/
public function getPostByCategoryId($category_id)
{
$result = $this->where([
['category_id', $category_id],
['delete_flg', 0],
])
->get();
return $result;
}
/**
* ユーザーIDに紐づいた投稿リストを全て取得する
*
* @param int $user_id ユーザーID
* @return Post
*/
public function getAllPostsByUserId($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 0],
])
->with('category')
->orderBy('updated_at', 'DESC')
->get();
return $result;
}
/**
* 下書き保存=>publish_flg=0
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToSaveDraft($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 公開=>publish_flg=1
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 予約公開=>publish_flg=2
* リクエストされたデータをpostsテーブルにinsertする
*
* @param int $user_id ログインユーザーID
* @param array $request リクエストデータ
* @return object $result App\Models\Post
*/
public function insertPostToReservationRelease($user_id, $request)
{
$result = $this->create([
'user_id' => $user_id,
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
'view_counter' => 0,
'favorite_counter' => 0,
'delete_flg' => 0,
]);
return $result;
}
/**
* 投稿IDをもとにpostsテーブルから一意の投稿データを取得
*
* @param int $post_id 投稿ID
* @return object $result App\Models\Post
*/
public function feachPostDateByPostId($post_id)
{
$result = $this->find($post_id);
return $result;
}
/**
* 記事の更新処理
* 下書き保存=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToSaveDraft($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 0,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開=>publish_flg=1
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 1,
]);
$result->save();
return $result;
}
/**
* 記事の更新処理
* 公開予約=>publish_flg=0
* リクエストされたデータをもとにpostデータを更新する
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function updatePostToReservationRelease($request, $post)
{
$result = $post->fill([
'category_id' => $request->category,
'title' => $request->title,
'body' => $request->body,
'publish_flg' => 2,
]);
$result->save();
return $result;
}
/**
* ゴミ箱一覧の記事を取得
*
* @param int $user_id ユーザーID
* @return object $result App\Models\Post
*/
public function getTrashPostLists($user_id)
{
$result = $this->where([
['user_id', $user_id],
['delete_flg', 1],
])
->get();
return $result;
}
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function moveTrashPostData($post)
{
$result = $post->fill([
'delete_flg' => 1
]);
$result->save();
return $result;
}
/**
* 記事の復元
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function restorePostData($post)
{
$result = $post->fill([
'delete_flg' => 0
]);
$result->save();
return $result;
}
/**
* 記事の削除
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function deletePostData($post)
{
$result = $post->delete();
return $result;
}
}
これで、ゴミ箱のdeleteをクリックした時に確認ダイアログ表示→OK押す→ゴミ箱から記事が消える→DB上からもデータが消えるの流れが作れました。
これでゴミ箱と記事の削除は終わりっす!
ただ、ちょっとこのままだと問題があります。個人差ではありますが、記事を復元した際に、ステータスがそのままなので、下書き保存にステータスをしておきます。
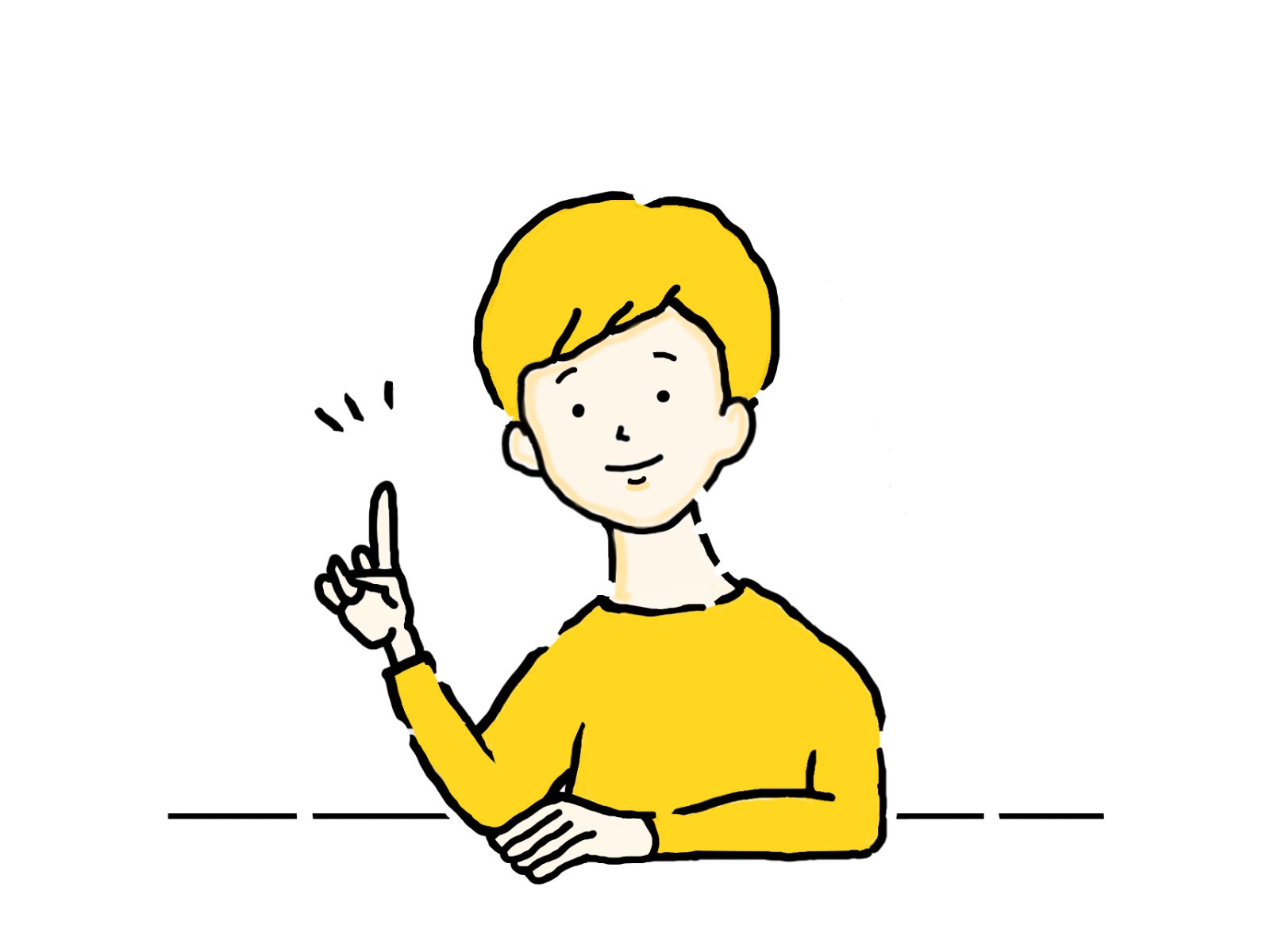
例えば、公開中の記事を何か問題あってゴミ箱に移動したけど、「やっぱ復元したい」ってなって復元した場合あるよね?
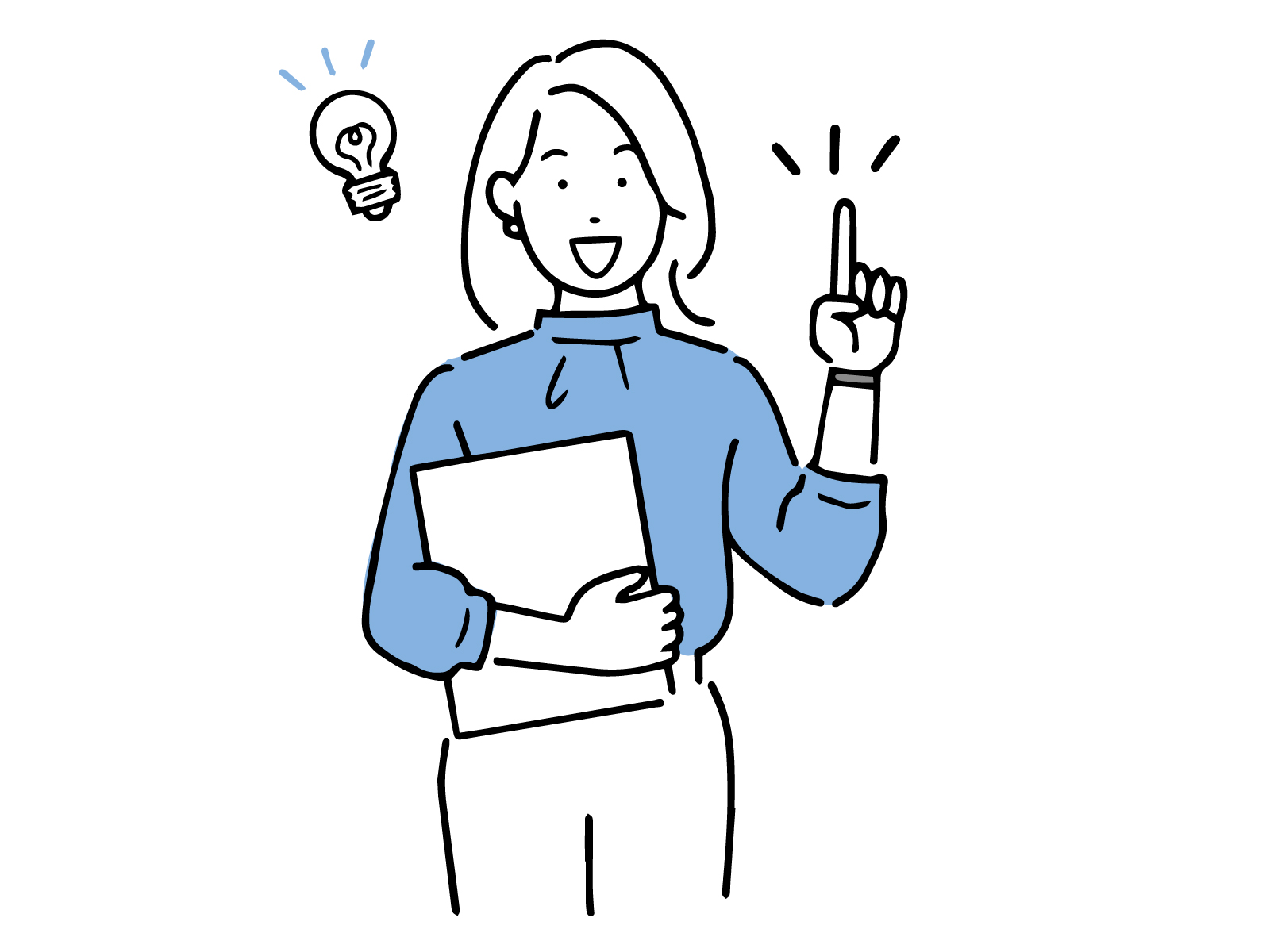
確かにあります!
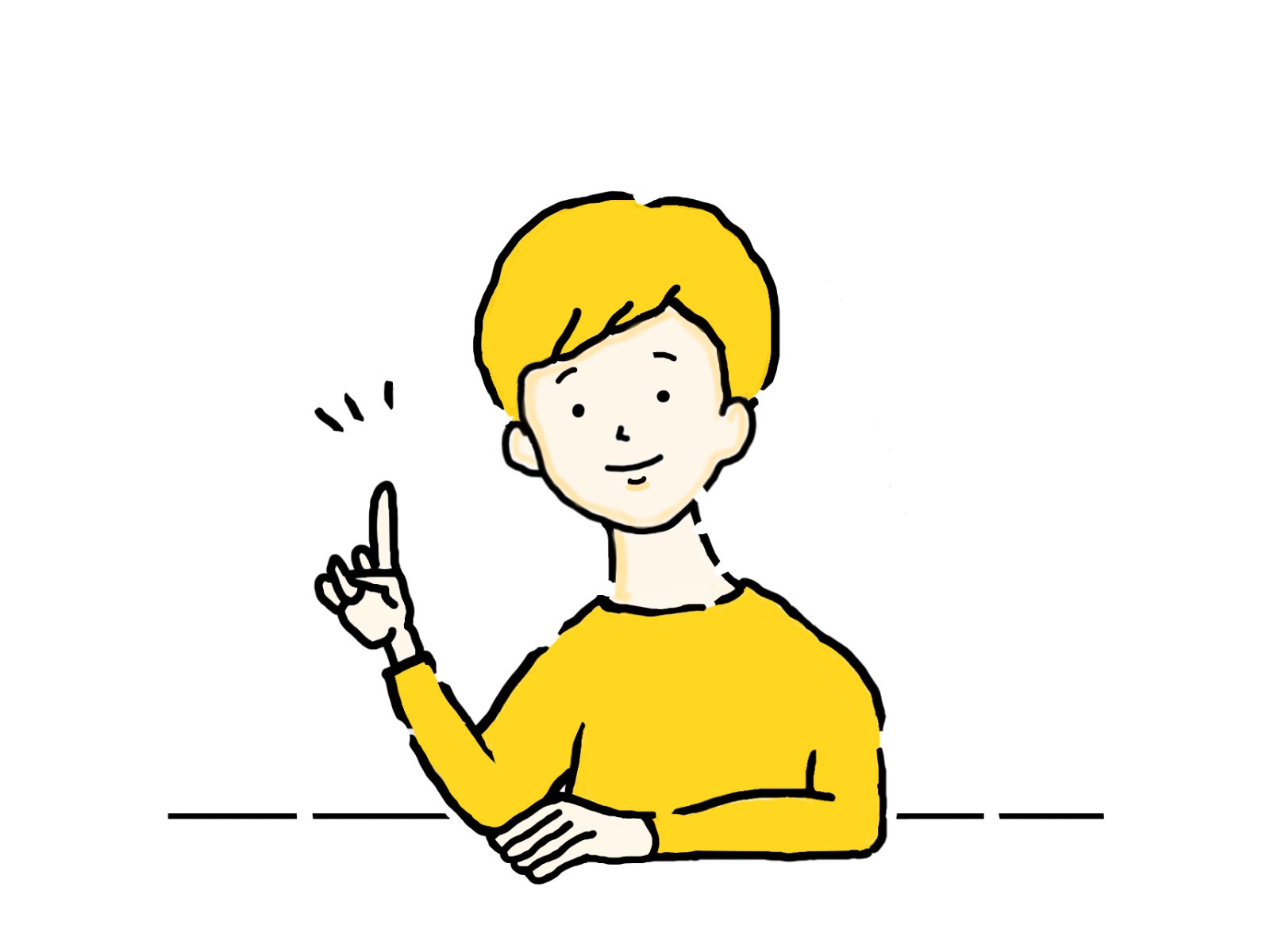
そんな時にゴミ箱から復元させた記事のステータスが公開中で復元されたら、もしかしたら良くない場合も考えられるケースありそうじゃない?
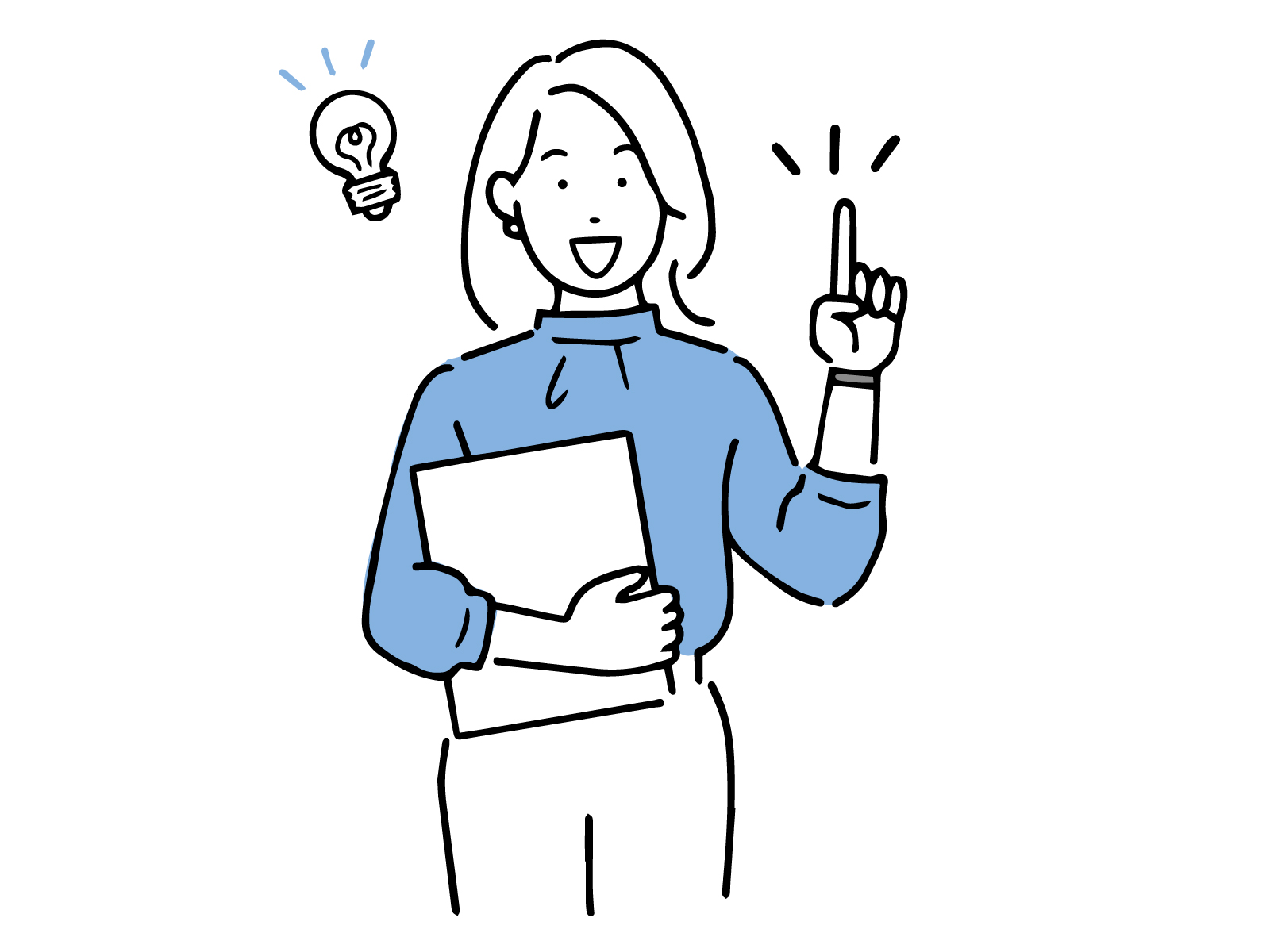
確かにあります!(といっておこう)
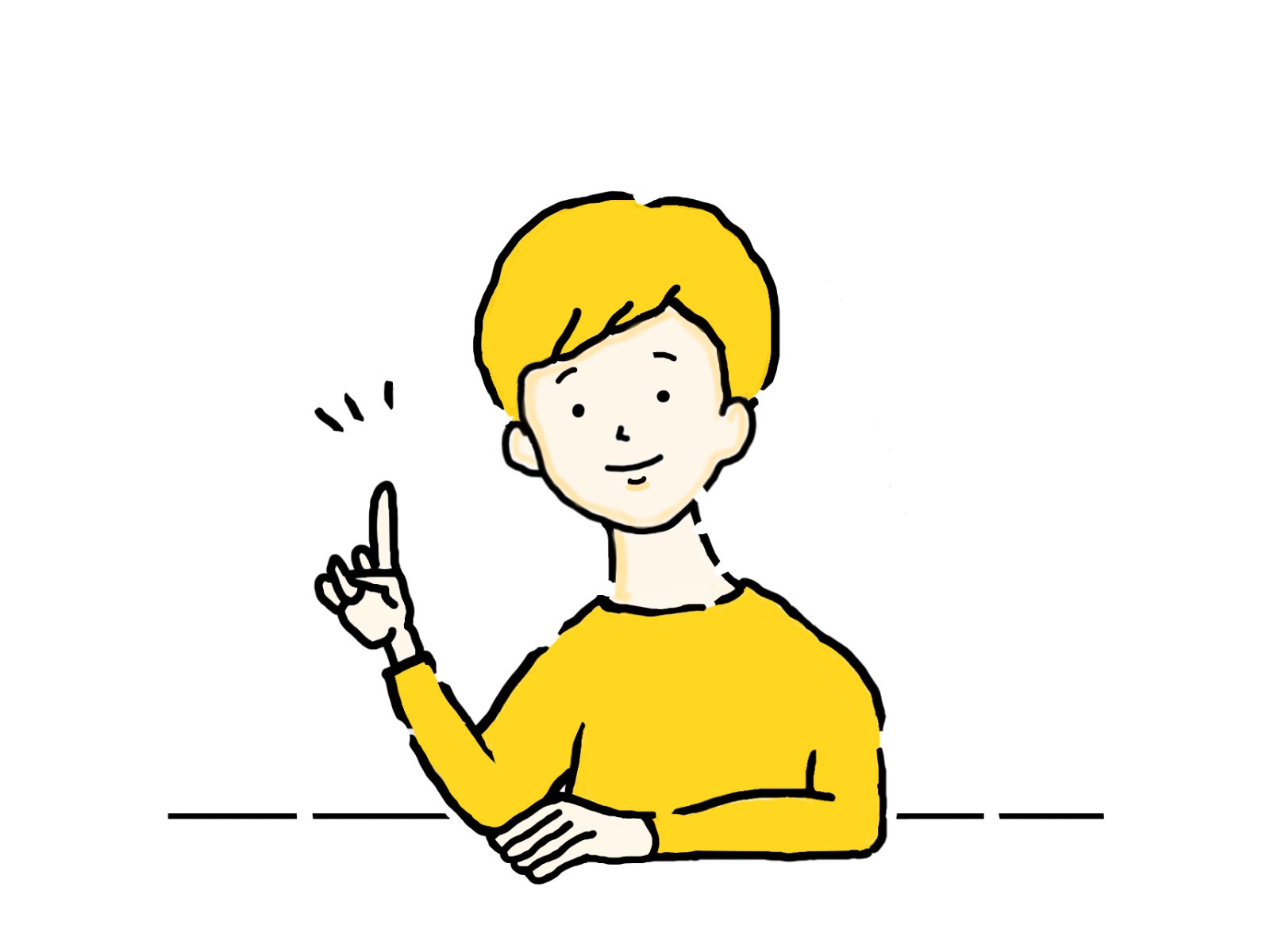
ゴミ箱に移動、復元する際にステータスを下書き保存にしておけば、ゴミ箱から復元する際にステータスは下書き保存になっていて後から編集して再度公開するなど都合がよさそうだったりすると思うんだよね〜
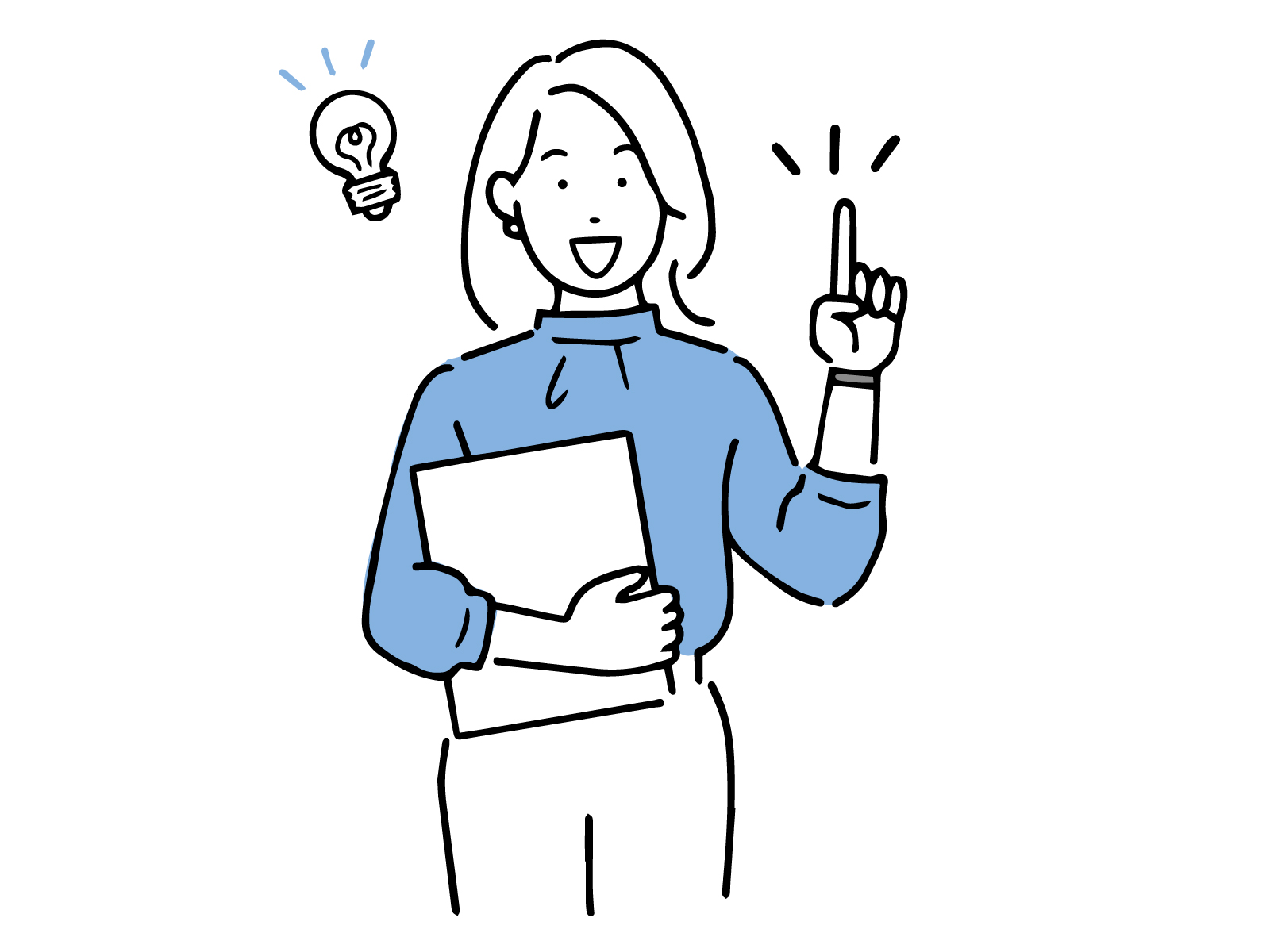
確かに!先輩すごい!(といっておこう)
/**
* 記事の論理削除(ゴミ箱に移動)
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function moveTrashPostData($post)
{
$result = $post->fill([
'publish_flg' => 0,
'delete_flg' => 1
]);
$result->save();
return $result;
}
/**
* 記事の復元
*
* @param array $post 投稿データ
* @return object $result App\Models\Post
*/
public function restorePostData($post)
{
$result = $post->fill([
'publish_flg' => 0,
'delete_flg' => 0
]);
$result->save();
return $result;
}
また、ちょっとしたリファクタリングをやります。Laravel9からルーティングにてコントローラーで処理をまとめることができるのでこちらやっていきましょう!
参考:Laravel9公式ルーティングのコントローラを参照
Laravel8とかだとコントローラーでまとめることはできないので注意が必要です。実際にどのように変わったかというと…
Laravel8
Route::get('/post/create', [PostController::class, 'create'])
Route::get('/post/hoge', [PostController::class, 'hoge'])
Route::get('/post/foo', [PostController::class, 'foo'])
....
と書いていくしかなかった。
Laravel9
// PostController::classの部分でまとめられる
Route::controller(PostController::class)->group(function() {
Route::get('/post/create', 'create')
Route::get('/post/hoge', 'hoge')
Route::get('/post/foo', 'foo')
});
いちいちPostController::classを書かなくても良くなったので便利!
Laravel6とかだと少しルーティングの書き方が違うので、Laravel6公式ルーティングを参照ください。
それではルーティングをリファクタリングをすると以下のようになります。
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TopController;
use App\Http\Controllers\User\PostController;
use App\Http\Controllers\User\TrashController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/dashboard', function () {
return view('dashboard');
})->middleware(['auth'])->name('dashboard');
require __DIR__.'/auth.php';
// 総合トップ
Route::controller(TopController::class)->group(function() {
// 総合トップ画面
Route::get('/', 'top')
->name('top');
// 総合トップ記事詳細画面
Route::get('/article/{post_id}', 'articleShow')
->name('top.article.show');
// 総合トップカテゴリーごとの記事一覧
Route::get('/article/category/{category_id}', 'articleCategory')
->name('top.article.category');
});
// マイページ投稿関係
Route::controller(PostController::class)->group(function() {
// マイページトップ・投稿
Route::get('/user/{id}/index', 'index')
->name('user.index');
// 投稿登録画面
Route::get('/post/create', 'create')
->name('post.create');
// 投稿登録処理
Route::post('/post/store', 'store')
->name('post.store');
// 投稿詳細
Route::get('/post/show/{post_id}', 'show')
->name('post.show');
// 記事編集
Route::get('/post/edit/{post_id}', 'edit')
->name('post.edit');
// 記事更新
Route::post('/post/edit/{post_id}', 'update')
->name('post.update');
});
// ゴミ箱関係
Route::controller(TrashController::class)->group(function() {
// 記事のゴミ箱
Route::get('/post/trash', 'trashList')
->name('post.trash');
// 記事論理削除(ゴミ箱に移動)
Route::post('/post/trash/{post_id}', 'moveTrash')
->name('post.move.trash');
// 記事の復元(ゴミ箱から投稿リストに戻す)
Route::post('/post/restore/{post_id}', 'restore')
->name('post.restore');
// 記事を完全に削除
Route::post('/post/delete/{post_id}', 'delete')
->name('post.delete');
});
はい!これで本当にこの章の記事は終わりです。
次回は、マイページのサイドバーに追加で公開中を作成&下書き保存や予約公開をクリックした際に、下書き保存の記事一覧や公開中の記事一覧を表示するようにしましょう。
あと、記事の作成や更新、削除などをしたときにフラッシュメッセージを表示するようにもするので、よりユーザーが使いやすく改良していきます。
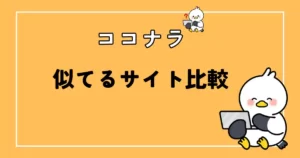
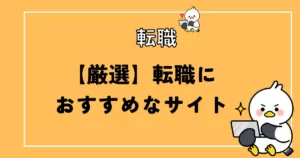
休日で空いた時間の暇つぶしを探せるアプリを公開しています。
コメント