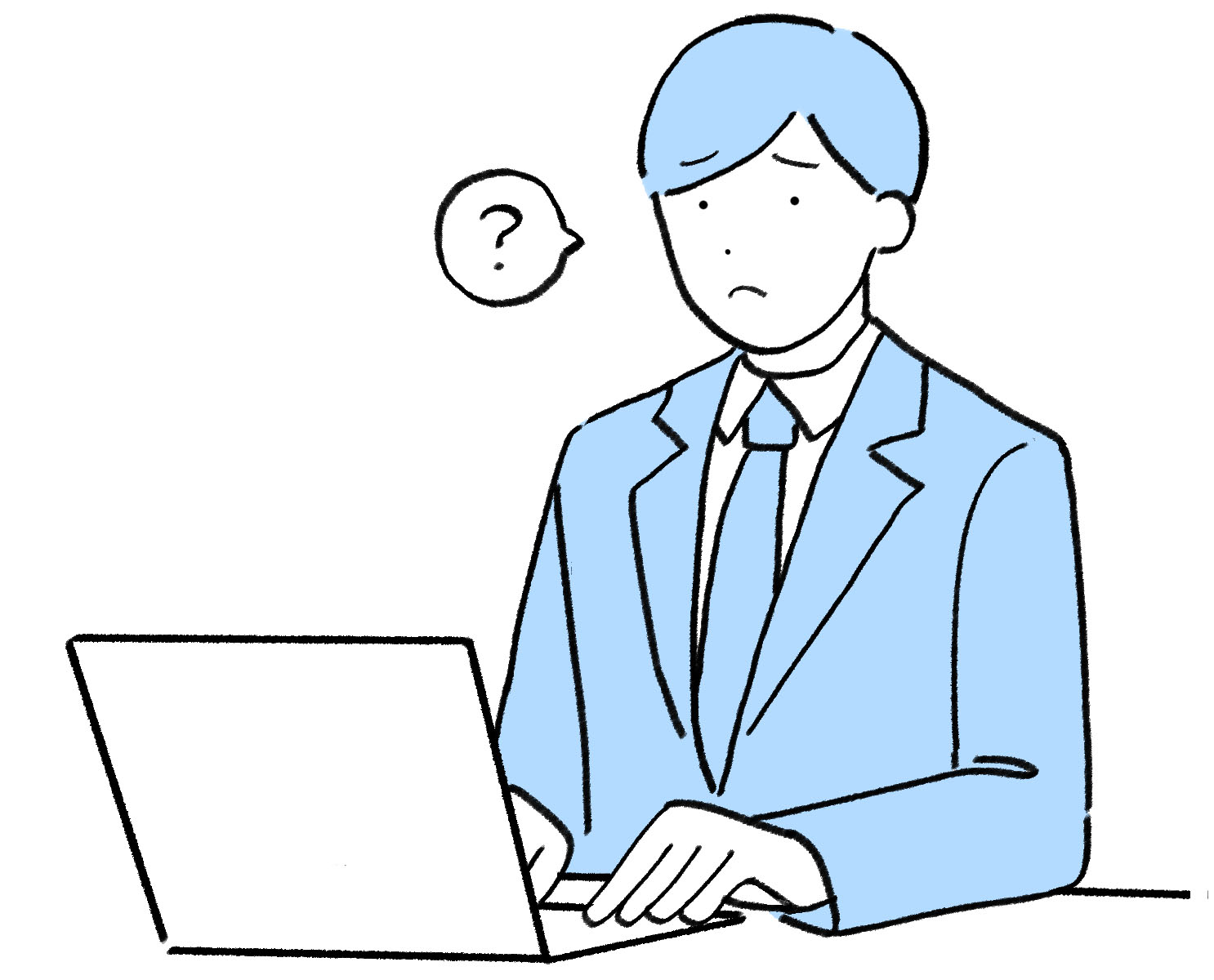
cakePHP4でTable class for alias テーブル名 could not be found.のエラーが出た…
こんな疑問を解決します。
【開発環境】
PHP8
cakePHP4
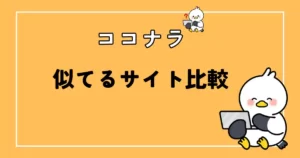
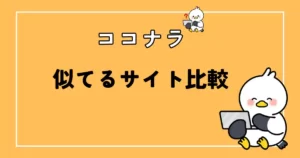
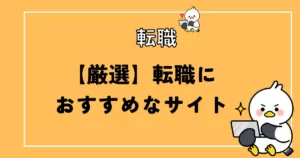
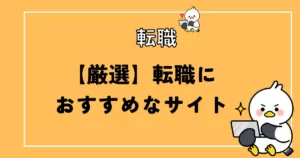
休日で空いた時間の暇つぶしを探せるアプリを公開しています。
結論
コントローラーに対応するモデルが正しくできていないことが原因。
ItemsControllerを作成していれば、bin/cake bake model itemsでコントローラーに対応するモデルを作ることが重要。
もし、テーブルとモデルとコントローラーがあっていそうだなと思っても、cakePHP4の命名規則に則っていなくて紐づいていないのが原因です。
エラーが発生した内容
私の環境でエラーが起きたのは、以下のようにコントローラーでTableRegistry::getを用いて、テーブルの情報が取れるか確認しようとした時です。
<?php
declare(strict_types=1);
namespace App\Controller;
use Cake\ORM\TableRegistry;
/**
* Items Controller
*
* @method \App\Model\Entity\Item[]|\Cake\Datasource\ResultSetInterface paginate($object = null, array $settings = [])
*/
class ItemsController extends AppController
{
public function initialize(): void
{
parent::initialize();
//itemsのDBを指定
$this->items = TableRegistry::get('Items');
dd($this->items);
}
public function index()
{
}
}
ItemsControllerでテーブルを指定し、念の為デバッグでデータが取れるか確認しようとしたところTable class for alias items could not be found.が発生しました。
エラーが発生したときに対応したこと
エラー原因を読んでいくと、
Table class for alias items could not be found.とあり、
日本語に訳すと、「itemsテーブルが見つかりません」みたいな意味になります。
なので、itemsテーブルはあるか?ItemsControllerに対応するモデルがちゃんとできているか確認します。
すると、
- itemsテーブルは作成ずみ
ただ、ItemsControllerに対応するモデルで、Model > Entity > item.phpはあるが、Model > Table > itemsTable.phpがないことが判明しました。
よって、itemsのモデルを再作成します。
※Model > Entity > item.phpは削除してから以下を実行
bin/cake bake model items
これで、Model > Entity > item.phpはあるが、Model > Table > itemsTable.phpが作成されました。
<?php
declare(strict_types=1);
namespace App\Model\Entity;
use Cake\ORM\Entity;
/**
* Item Entity
*
* @property int $id
* @property string $name
* @property int|null $price
* @property string|null $content
* @property bool $published
* @property \Cake\I18n\FrozenTime $created_at
* @property \Cake\I18n\FrozenTime|null $updated_at
*/
class Item extends Entity
{
/**
* Fields that can be mass assigned using newEntity() or patchEntity().
*
* Note that when '*' is set to true, this allows all unspecified fields to
* be mass assigned. For security purposes, it is advised to set '*' to false
* (or remove it), and explicitly make individual fields accessible as needed.
*
* @var array
*/
protected $_accessible = [
'name' => true,
'price' => true,
'content' => true,
'published' => true,
'created_at' => true,
'updated_at' => true,
];
}
以下、$this->setTable(‘items’);となっており、itemsテーブルをセットしていることも確認できます。
<?php
declare(strict_types=1);
namespace App\Model\Table;
use Cake\ORM\Query;
use Cake\ORM\RulesChecker;
use Cake\ORM\Table;
use Cake\Validation\Validator;
/**
* Items Model
*
* @method \App\Model\Entity\Item newEmptyEntity()
* @method \App\Model\Entity\Item newEntity(array $data, array $options = [])
* @method \App\Model\Entity\Item[] newEntities(array $data, array $options = [])
* @method \App\Model\Entity\Item get($primaryKey, $options = [])
* @method \App\Model\Entity\Item findOrCreate($search, ?callable $callback = null, $options = [])
* @method \App\Model\Entity\Item patchEntity(\Cake\Datasource\EntityInterface $entity, array $data, array $options = [])
* @method \App\Model\Entity\Item[] patchEntities(iterable $entities, array $data, array $options = [])
* @method \App\Model\Entity\Item|false save(\Cake\Datasource\EntityInterface $entity, $options = [])
* @method \App\Model\Entity\Item saveOrFail(\Cake\Datasource\EntityInterface $entity, $options = [])
* @method \App\Model\Entity\Item[]|\Cake\Datasource\ResultSetInterface|false saveMany(iterable $entities, $options = [])
* @method \App\Model\Entity\Item[]|\Cake\Datasource\ResultSetInterface saveManyOrFail(iterable $entities, $options = [])
* @method \App\Model\Entity\Item[]|\Cake\Datasource\ResultSetInterface|false deleteMany(iterable $entities, $options = [])
* @method \App\Model\Entity\Item[]|\Cake\Datasource\ResultSetInterface deleteManyOrFail(iterable $entities, $options = [])
*/
class ItemsTable extends Table
{
/**
* Initialize method
*
* @param array $config The configuration for the Table.
* @return void
*/
public function initialize(array $config): void
{
parent::initialize($config);
$this->setTable('items');
$this->setDisplayField('name');
$this->setPrimaryKey('id');
}
/**
* Default validation rules.
*
* @param \Cake\Validation\Validator $validator Validator instance.
* @return \Cake\Validation\Validator
*/
public function validationDefault(Validator $validator): Validator
{
$validator
->integer('id')
->allowEmptyString('id', null, 'create');
$validator
->scalar('name')
->maxLength('name', 255)
->requirePresence('name', 'create')
->notEmptyString('name');
$validator
->allowEmptyString('price');
$validator
->scalar('content')
->allowEmptyString('content');
$validator
->boolean('published')
->notEmptyString('published');
$validator
->dateTime('created_at')
->requirePresence('created_at', 'create')
->notEmptyDateTime('created_at');
$validator
->dateTime('updated_at')
->allowEmptyDateTime('updated_at');
return $validator;
}
}
これで、エラーは解消されました。
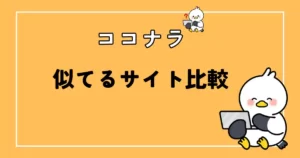
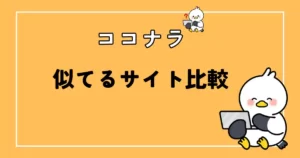
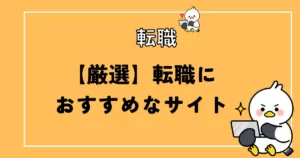
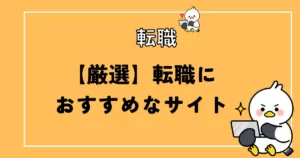
休日で空いた時間の暇つぶしを探せるアプリを公開しています。
コメント