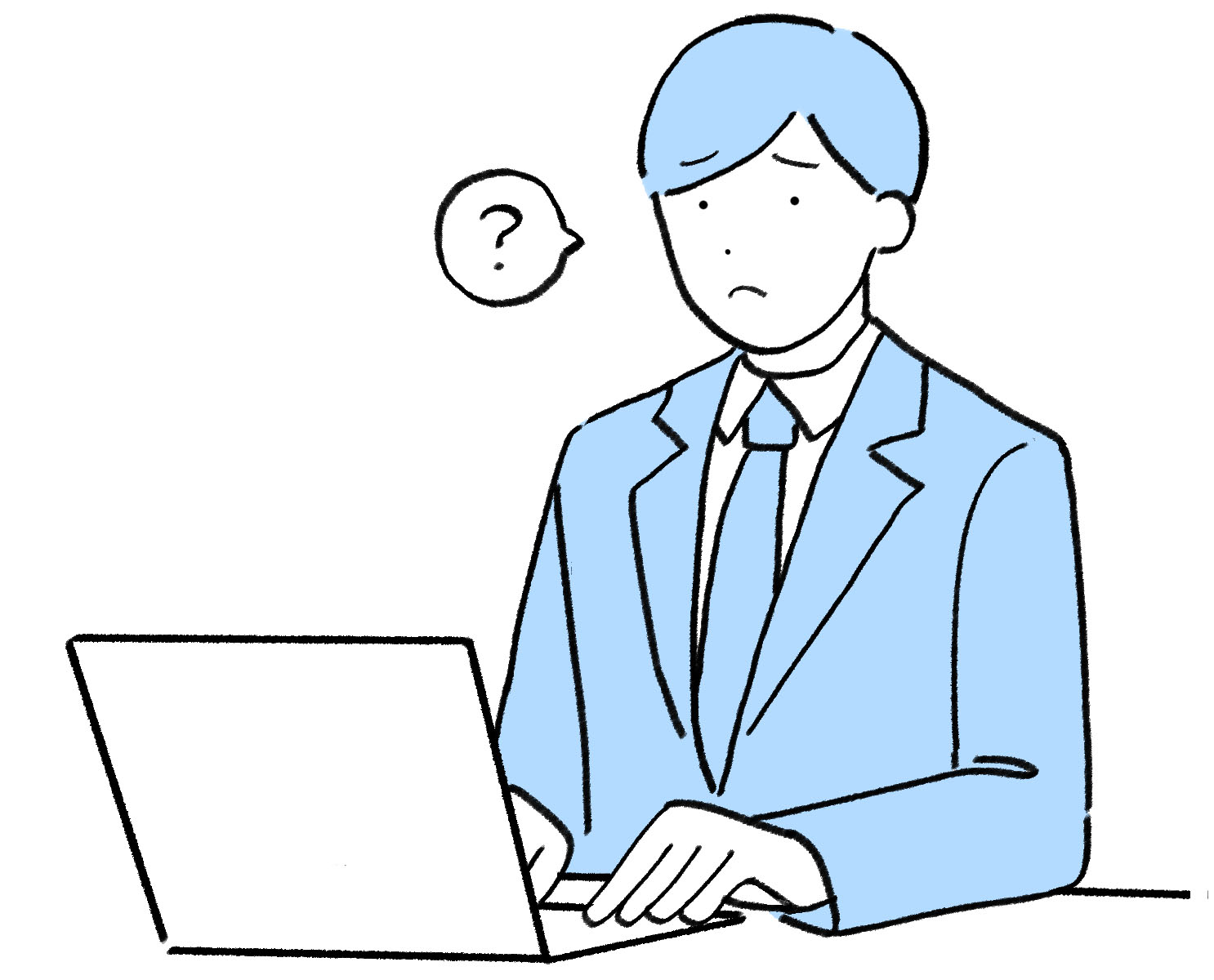
cakePHP4でseeder(シーダー)を実行するにはどうしたらいいんだろう…
こんな疑問を解決します。
マイグレーションでテーブルを作成し、データを1件1件入れていくのもいいです。
が、seeder(シーダー)を活用すればもしテーブルのデータが消えてもseederファイルを実行するだけでデータを投入できます。
【開発環境】
PHP8.1
cakePHP4
事前にitemsテーブルを作成しておきましょう。↓itemsテーブルの中身
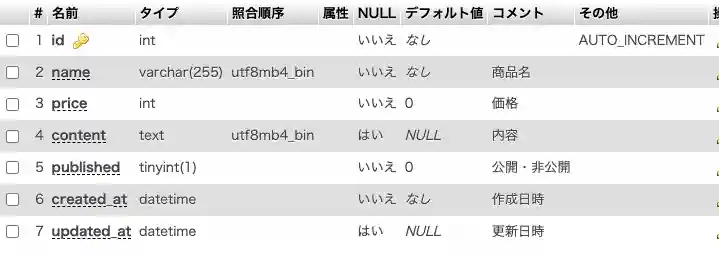
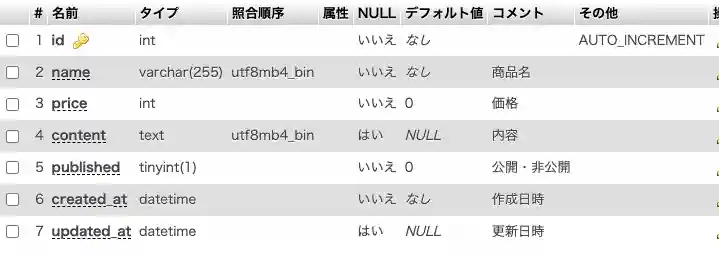
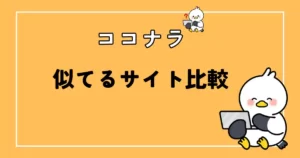
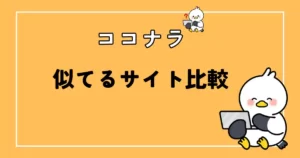
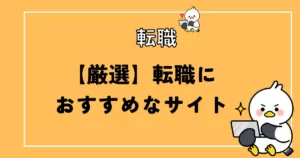
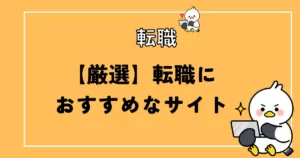
休日で空いた時間の暇つぶしを探せるアプリを公開しています。
cakePHP4でseederファイルを作成する
bakeコマンドでseederファイルを作成しましょう。
$ bin/cake bake seed シーダーファイル名
config > Seeds > にItemsSeed.phpが作成されます。
※シーダーファイル名は、できるだけテーブル名に合わせた方がいいです。
bin/cake bake seed Items
Creating file /var/www/html/config/Seeds/ItemsSeed.php
Wrote `/var/www/html/config/Seeds/ItemsSeed.php`
seederファイルを編集します。
<?php
declare(strict_types=1);
use Migrations\AbstractSeed;
/**
* Items seed.
*/
class ItemsSeed extends AbstractSeed
{
/**
* Run Method.
*
* Write your database seeder using this method.
*
* More information on writing seeds is available here:
* https://book.cakephp.org/phinx/0/en/seeding.html
*
* @return void
*/
public function run()
{
// itemsテーブルを指定
$table = $this->table('items');
// テーブルのデータを空にする
$table->truncate();
// データ
$data = [
[
'id' => 1,
'name' => 'iphone',
'price' => 120000,
'published' => 1,
'created_at' => '2022-03-07 12:00:00',
'updated_at' => null,
],
[
'id' => 2,
'name' => 'Mac',
'price' => 200000,
'published' => 1,
'created_at' => '2022-03-07 13:00:00',
'updated_at' => null,
],
[
'id' => 3,
'name' => 'book',
'price' => 2000,
'published' => 0,
'created_at' => '2022-03-07 14:00:00',
'updated_at' => null,
],
];
// itemsテーブルにデータをインサート
$table->insert($data)->save();
}
}
seederファイルを実行します。
$ bin/cake migrations seed
using migration paths
- /var/www/html/config/Migrations
using seed paths
- /var/www/html/config/Seeds
using migration paths
- /var/www/html/config/Migrations
using seed paths
- /var/www/html/config/Seeds
using environment default
using adapter mysql
using database cakephp4_tests
== ItemsSeed: seeding
== ItemsSeed: seeded 0.1615s
All Done. Took 0.1872s
成功しました。
itemsテーブルを確認すると、しっかりとデータが入っていますね。
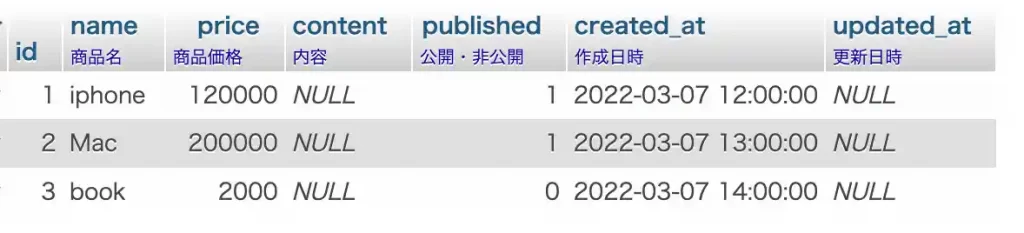
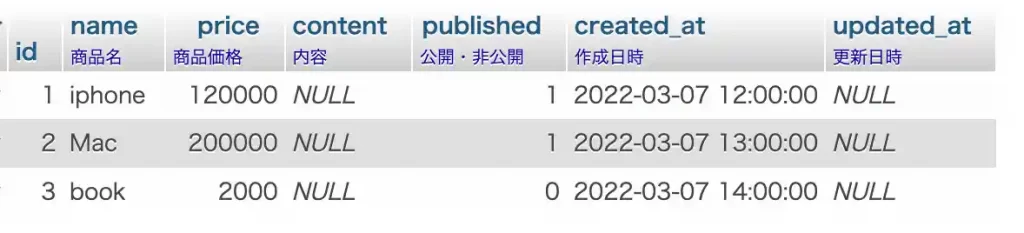
seederファイル名とテーブル名が異なる場合
以下のように書きます。
$ bin/cake bake seed 任意のseederファイル名 --table テーブル名
ex.
$ bin/cake bake seed ItemData --table items
これで、config > Seeds > にItemData.phpができますが、指定するテーブルはitemsテーブルになります。
もし、–table itemsをつけなかった場合、指定するテーブルがitem_dataとなり、「そんなテーブルねえからな!」と言われるでしょう。
–tableオプションをつけなかった場合でも、$table = $this->table(‘テーブル名’);とすれば大丈夫です。
seederファイルで外部キーがある場合の対応
外部キーがある場合、truncateを実行するとエラーになる場合があるようです。
なので、truncateする前にSET FOREIGN_KEY_CHECKS = 0を使うのがポイント。
※truncateした後は、SET FOREIGN_KEY_CHECKS = 1をはる。
<?php
declare(strict_types=1);
use Migrations\AbstractSeed;
/**
* Items seed.
*/
class ItemsSeed extends AbstractSeed
{
/**
* Run Method.
*
* Write your database seeder using this method.
*
* More information on writing seeds is available here:
* https://book.cakephp.org/phinx/0/en/seeding.html
*
* @return void
*/
public function run()
{
// itemsテーブルを指定
$table = $this->table('items');
// 外部キー制約取消し
$table->execute('SET FOREIGN_KEY_CHECKS = 0');
// テーブルのデータを空にする
$table->truncate();
// 外部キー制約取消し
$table->execute('SET FOREIGN_KEY_CHECKS = 1');
// データ
$data = [
[
'id' => 1,
'name' => 'iphone',
'price' => 120000,
'published' => 1,
'created_at' => '2022-03-07 12:00:00',
'updated_at' => null,
],
[
'id' => 2,
'name' => 'Mac',
'price' => 200000,
'published' => 1,
'created_at' => '2022-03-07 13:00:00',
'updated_at' => null,
],
[
'id' => 3,
'name' => 'book',
'price' => 2000,
'published' => 0,
'created_at' => '2022-03-07 14:00:00',
'updated_at' => null,
],
];
// itemsテーブルにデータをインサート
$table->insert($data)->save();
}
}
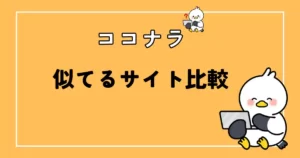
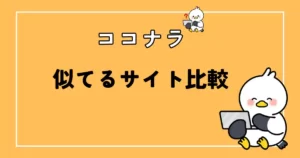
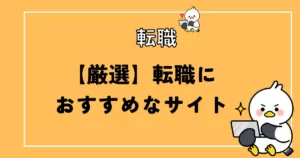
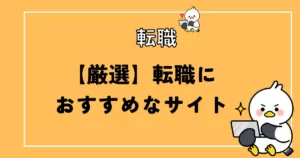
休日で空いた時間の暇つぶしを探せるアプリを公開しています。
コメント